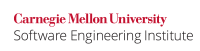
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <new>
#include <type_traits>
void f() {
char c; // Used elsewhere in the function
std::aligned_storage<sizeof(long), alignof(long)>::type buffer;
long *lp = ::new (&buffer) long;
// ...
} |
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <new>
void f() {
char c; // Used elsewhere in the function
alignas(long) unsigned char buffer[sizeof(long)];
long *lp = ::new (buffer) long;
// ...
} |
...
The amount of overhead required by array new expressions is unspecified but should be documented by quality implementations. The following snippet outlines an example compliant solution that's portable to the Clang and GNU GCC compilers. Porting it to other implementations requires adding similar conditional definitions of the Overhead
overhead
constant. When an implementation does not document the overhead, assuming it's at least as large as twice the size of sizeof(size_t)
should typically be safe. To verify that the assumption is, in fact, safe, the compliant solution defines an overload of the placement operator new that accepts the buffer size as a third argument and verifies that it is at least as large as the total amount of storage required.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <cassert> #include <cstddef> #if __clang__ || __GNUG__ const size_t Overheadoverhead = sizeof(size_t); #else const size_t Overheadoverhead = 2 * sizeof(size_t); #endif struct S { void *p; S (); ~S (); }; void* operator new[] (size_t n, void *p, size_t bufsize) { assert (n <= bufsize); // alternatively, throw an exception return p; } void f() { const size_t Nn = 32; alignas(S) unsigned char buffer[sizeof(S) * Nn + Overheadoverhead]; S *sp = new (buffer, sizeof buffer) S [Nn]; // ... // Destroy elements of the array. for (size_t i = 0; i != Nn; ++i) sp[i].~S (); } |
Risk Assessment
Passing improperly aligned pointers or pointers to insufficient storage to placement new expressions can result in undefined behavior, including buffer overflow and abnormal termination.
...