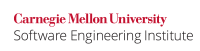
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <cstring> #include <iostream> class C { int ScalingFactorscalingFactor; int OtherDataotherData; public: C() : ScalingFactorscalingFactor(1) {} void SetOtherDataset_other_data(int Ii); int f(int i) { return i / ScalingFactorscalingFactor; } // ... }; void f() { C c; // ... Code that mutates c ... // Reinitialize c to its default state std::memset(&c, 0, sizeof(C)); std::cout << c.f(100) << std::endl; } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <iostream> #include <utility> class C { int ScalingFactorscalingFactor; int OtherDataotherData; public: C() : ScalingFactorscalingFactor(1) {} void SetOtherDataset_other_data(int Ii); int f(int i) { return i / ScalingFactorscalingFactor; } // ... }; template <typename T> T& clear(T &o) { using std::swap; T empty; swap(o, empty); return o; } void f() { C c; // ... Code that mutates c ... // Reinitialize c to its default state clear(c); std::cout << c.f(100) << std::endl; } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <cstring> class C { int *Ii; public: C() : Ii(nullptr) {} ~C() { delete Ii; } // ... }; void f(C &c1) { C c2; std::memcpy(&c2, &c1, sizeof(C)); } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
class C { int *Ii; public: C() : Ii(nullptr) {} ~C() { delete Ii; } C &operator=(const C &RHSrhs) noexcept(false) { if (this != &RHSrhs) { int *Oo = nullptr; if (RHSrhs.Ii) { Oo = new int; *Oo = *RHSrhs.Ii; } // Does not modify this unless allocation succeeds. delete Ii; Ii = O; o; } return *this; } // ... }; void f(C &c1) { C c2 = c1; } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <cstring> class C { int Ii; public: virtual void f(); // ... }; void f(C &c1, C &c2) { if (!std::memcmp(&c1, &c2, sizeof(C))) { // ... } } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
class C { int Ii; public: virtual void f(); bool operator==(const C &RHSrhs) const { return RHSrhs.Ii == Ii; } // ... }; void f(C &c1, C &c2) { if (c1 == c2) { // ... } } |
...