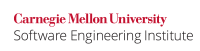
...
In this example, the function f()
is passed a const
char
pointer. It then typecasts the const
specification away and proceeds to modify the contents.
Code Block | ||||
---|---|---|---|---|
| ||||
void f(char const *str, int slen) { char *p = const_cast<char*>(str); int i; for (i = 0; i < slen && str[i]; i++) { if (str[i] != ' ') *p++ = str[i]; } } |
...
In this compliant solution, the function f
is passed a non-const
char
pointer. The calling function must ensure that the null-terminated byte string passed to the function is not const
by making a copy of the string or by other means.
Code Block | ||||
---|---|---|---|---|
| ||||
void f(char *str, int slen) { char *p = str; int i; for (i = 0; i < slen && str[i]; i++) { if (str[i] != ' ') *p++ = str[i]; } } |
...
In this example, a const
int
array vals
is declared, and then its content is modified by calling memset
with the function, leading to values of 0 in the vals
array.
Code Block | ||||
---|---|---|---|---|
| ||||
int const vals[] = {3, 4, 5}; memset((int*) vals, 0, sizeof(vals)); |
...
If the intention is to allow the array values to be modified, do not declare the array as const
.
Code Block | ||||
---|---|---|---|---|
| ||||
int vals[] = {3, 4, 5}; memset(vals, 0, sizeof(vals)); |
...