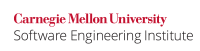
...
In this compliant solution, the copy assignment operator provides the strong exception safety guarantee. The function allocates new storage for the copy before changing the state of the object. Only after the allocation succeeds does the function proceed to change the state of the object. In addition, by copying the array to the newly allocated storage before deallocating the existing array, the function avoids the test for self-assignment, which improves the performance of the code in the common case [Sutter 2004].
Code Block | ||||
---|---|---|---|---|
| ||||
#include <cstring> class IntArray { int *array; std::size_t nElems; public: // ... ~IntArray() { delete[] array; } IntArray(const IntArray& that); // nontrivial copy constructor IntArray& operator=(const IntArray &rhs) { int *tmp = nullptr; if (rhs.nElems) { tmp = new int[rhs.nElems]; std::memcpy(tmp, rhs.array, rhs.nElems * sizeof(*array)); } delete[] array; array = tmp; nElems = rhs.nElems; return *this; } // ... }; |
...
[ISO/IEC 14882-2014] | Clause 15, "Exception Handling" |
[Stroustrup 01] | |
[Sutter 00] | |
[Sutter 01] | |
[Sutter 04] | 55. "Prefer the canonical form of assignment." |
...