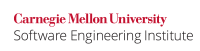
The C++ Standard, [basic.types], paragraph 9 [ISO/IEC 14882-2014], states the following:
The object representation of an object of type
T
is the sequence of Nunsigned char
objects taken up by the object of typeT
, where N equalssizeof(T)
. The value representation of an object is the set of bits that hold the value of typeT
.
The narrow character types (char
, signed char
, and unsigned char
)—as well as some other integral types on specific platforms—have an object representation that consists solely of the bits from the object's value representation. For such types, accessing any of the bits of the value representation is well-defined behavior. This form of object representation allows a programmer to access and modify an object solely based on its bit representation, such as by calling std::memcmp()
on its object representation.
Other types, such as classes, may not have an object representation composed solely of the bits from the object's value representation. For instance, classes may have bit-field data members, padding inserted between data members, a vtable to support virtual method dispatch, or data members declared with different access privileges. For such types, accessing bits of the object representation that are not part of the object's value representation may result in undefined behavior depending on how those bits are accessed.
...
In this compliant solution, S
overloads operator==()
to perform a comparison of the value representation of the object:.
Code Block | ||||
---|---|---|---|---|
| ||||
struct S { unsigned char buffType; int size; friend bool operator==(const S &lhs, const S &rhs) { return lhs.buffType == rhs.buffType && lhs.size == rhs.size; } }; void f(const S &s1, const S &s2) { if (s1 == s2) { // ... } } |
...
In this compliant solution, the data members of S
are cleared explicitly instead of calling std::memset()
:.
Code Block | ||||
---|---|---|---|---|
| ||||
struct S { int i, j, k; // ... virtual void f(); void clear() { i = j = k = 0; } }; void f() { S *s = new S; // ... s->clear(); // ... s->f(); // ok } |
...