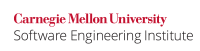
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <cassert> #include <cstddef> #if __clang__ || __GNUG__ const size_t overhead = sizeof(size_t); #else const size_t overhead = 2 * sizeof(size_t); #endif struct S { void *p; S (); ~S (); }; void* operator new[] (size_t n, void *p, size_t bufsize) { assert (n <= bufsize); // alternatively, throw an exception return p; } void f() { const size_t n = 32; alignas(S) unsigned char buffer[sizeof(S) * n + overhead]; S *sp = new (buffer, sizeof buffer) S[n]; // ... // Destroy elements of the array. for (size_t i = 0; i != n; ++i) { sp[i].~S (); } } |
Risk Assessment
Passing improperly aligned pointers or pointers to insufficient storage to placement new
expressions can result in undefined behavior, including buffer overflow and abnormal termination.
...