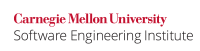
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <cstring>
class C {
int *i;
public:
C() : i(nullptr) {}
~C() { delete i; }
void set(int val) {
if (i) { delete i; }
i = new int{val};
}
// ...
};
void f(C &c1) {
C c2;
std::memcpy(&c2, &c1, sizeof(C));
} |
...
Code Block | ||||
---|---|---|---|---|
| ||||
class C { int *i; public: C() : i(nullptr) {} ~C() { delete i; } void set(int val) { if (i) { delete i; } i = new int{val}; } C &operator=(const C &rhs) noexcept(false) { if (this != &rhs) { int *o = nullptr; if (rhs.i) { o = new int; *o = *rhs.i; } // Does not modify this unless allocation succeeds. delete i; i = o; } return *this; } // ... }; void f(C &c1) { C c2 = c1; } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <cstring> class C { int i; public: virtual void f(); // ... }; void f(C &c1, C &c2) { if (!std::memcmp(&c1, &c2, sizeof(C))) { // ... } } |
Because a vtable is not part of an object's value representation, comparing it with std::memcmp()
is also a violation of EXP62-CPP. Do not access the bits of an object representation that are not part of the object's value representation.
Compliant Solution
In this compliant solution, C
defines an equality operator that is used instead of calling std::memcmp()
. This solution ensures that only the value representation of the objects is considered when performing the comparison.
...