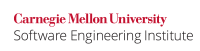
...
In this compliant solution, the parameter pos
is declared as size_t
, which prevents passing the passing of negative arguments.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <cstddef> void insert_in_table(int *table, std::size_t tableSize, std::size_t pos, int value) { if (pos >= tableSize) { // Handle error return; } table[pos] = value; } |
...
In this compliant solution, the parameter pos
is declared as size_t
, which ensures that the comparison expression will fail when a large, positive value (converted from a negative argument) is given:.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <vector> void insert_in_table(std::vector<int> &table, std::size_t pos, int value) { if (pos >= table.size()) { // Handle error return; } table[pos] = value; } |
...
This compliant solution tests for iterator validity before attempting to dereference b
:.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <iterator> template <typename ForwardIterator> void f_imp(ForwardIterator b, ForwardIterator e, int val, std::forward_iterator_tag) { while (b != e) { *b++ = val; } } template <typename ForwardIterator> void f(ForwardIterator b, ForwardIterator e, int val) { typename std::iterator_traits<ForwardIterator>::iterator_category cat; f_imp(b, e, val, cat); } |
...
[ISO/IEC 14882-2014] | Clause 23, "Containers Library" |
[ISO/IEC TR 24772-2013] | Boundary Beginning Violation [XYX] Wrap-around Around Error [XYY] Unchecked Array Indexing [XYZ] |
[Viega 05] | Section 5.2.13, "Unchecked Array Indexing" |
...