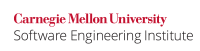
...
Code Block |
---|
#include <cstdlib> void f(int *int_list, size_t count) { std::qsort(int_list, count, sizeof(int), [](const void *lhs, const void *rhs) -> int { return reinterpret_cast<const int *>(lhs) < reinterpret_cast<const int *>(rhs); }); } |
Noncompliant Code Example
In this noncompliant code example, the call_java_fn_ptr()
function expects to receive a function pointer with "java"
language linkage because that function pointer will be used by a Java interpreter to call back into the C++ code. However, the function is given a pointer with "C++"
language linkage instead, resulting in undefined behavior when the interpreter attempts to call the function pointer. This code should be ill-formed because the type of callback_func()
is different than the type java_callback
, but due to common implementation divergence from the C++ Standard, some compilers may incorrectly accept this code without issuing a diagnostic.
Code Block | ||||
---|---|---|---|---|
| ||||
extern "java" typedef void (*java_callback)(int); extern void call_java_fn_ptr(java_callback callback); void callback_func(int); void f() { call_java_fn_ptr(callback_func); } |
Compliant Solution
In this compliant solution, the callback_func()
function is given "java"
language linkage to match the language linkage for java_callback
.
Code Block | ||||
---|---|---|---|---|
| ||||
extern "java" typedef void (*java_callback)(int); extern void call_java_fn_ptr(java_callback callback); extern "java" void callback_func(int); void f() { call_java_fn_ptr(callback_func); } |
Risk Assessment
Mismatched language linkage specifications generally do not create exploitable security vulnerabilities between the C and C++ language linkages. However, other language linkages exist where the undefined behavior is more likely to result in abnormal program execution, including exploitable vulnerabilities.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
EXP64EXP56-CPP | Low | Unlikely | Medium | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
|
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Bibliography
[ISO/IEC 14882-2014] | Subclause 5.2.2, "Function Call" Subclause 7.5, "Linkage Specifications" |
...