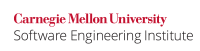
...
Compliant Solution (Array Templates)
Specialized function templates can be used to define functions that accept an array of a generic type {{ Wiki Markup T
}} of size {{n
}}. The compiler can perform template argument deduction for function templates to properly deduce both the type and size. This compliant solution defines a function template for a function {{clear()
}} that takes a template parameter {{array
\[
\]
}} of {{T
}} elements and an actual length parameter of type {{size_t
}}. This is not particularly useful yet, as we have already seen that passing an array with an explicit size parameter is a common (but error prone) idiom. However, you can also define a specialization of the function template that includes a template parameter {{n
}} of type {{size_t
}} in addition to the original type parameter. The inline function {{clear()
}} has one parameter: an array of type {{T
}} elements of fixed length {{n
}}.
Code Block | ||||
---|---|---|---|---|
| ||||
template <typename T> void clear(T array[], size_t n) { for (size_t i = 0; i < n; ++i) { array[i] = 0; } } template <typename T, size_t n> inline void clear(T (&array)[n]) { clear(array, n); } int int_array[12]; clear(int_array); // deduce T is int, and that n is 12 |
The function template and specialized function template can be used in a straightforward manner. This example declares an array of 12 integers named {{ Wiki Markup int_array
}} and invokes the {{clear()
}} function passing {{int_array
}} as an argument. The compiler matches this invocation to {{inline
void
clear(T
(&array)
\[n
\])
}} because this definition most closely matches the actual argument type of array of {{int
}}. The compiler deduces that the type {{T
}} is {{int
}} and that {{n
}} is 12, separating the size {{n
}} from the pointer to the array of {{int
}} before invoking the function template for {{clear()
}}. This use of specialized function templates guarantees that the {{clear()
}} function has the correct array size.
Noncompliant Code Example (Vectors)
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
ARR30-CPP | high | likely | high | P9 | L2 |
Automated Detection
The Coverity Prevent Version 5.0 NEGATIVE_RETUENS checker can detect where negative value is used to index an array in a read/write operation.
...
This rule appears in the C Secure Coding Standard as VOID Guarantee that array indices are within the valid range.
Bibliography
\[[ISO/IEC PDTR 24772|AA. Bibliography#ISO/IEC PDTR 24772]\] "XYX Boundary Beginning Violation," "XYY Wrap-around Error," and "XYZ Unchecked Array Indexing"
\ Wiki Markup
[[MITRE|AA. Bibliography#MITRE]\] [CWE ID 119|http://cwe.mitre.org/data/definitions/ 119.html], "Failure to Constrain Operations within the Bounds of a Memory Buffer"
\
[MITRE\] [CWE ID 129|http://cwe.mitre.org/data/definitions/ 129.html], "Improper Validation of Array Index"
\[
[Viega 05|AA. Bibliography#Viega 05] \] Section 5.2.13, "Unchecked array indexing"
...
ARR04-CPP. Assume responsibility for cleaning up data referenced by a container of pointers 06. Arrays and the STL (ARR) ARR31-CPP. Use consistent array notation across all source files