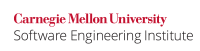
...
In this example, main()
does several useful work but does not catch any exceptions. Consequently, any exceptions thrown will call std::terminate()
, and might not destroy any objects owned by the program.
Code Block | ||||
---|---|---|---|---|
| ||||
int main(int argc, char** argv) { Object object; // might not get destroyed if exception thrown // do useful work return 0; } |
...
In this code example, all exceptions are caught, allowing normal termination, even in the face of unexpected errors (albeit with an exit status indicating that an error occurred).
Code Block | ||||
---|---|---|---|---|
| ||||
int main(int argc, char** argv) { Object object; int exit_status = EXIT_SUCCESS; try { // do useful work } catch (...) { exit_status = EXIT_FAILURE; } return exit_status; // object gets destroyed here } |
...
An alternative is to wrap all of main()
's functionality inside a try-catch block and catch and handle exceptions by exiting with a status indicating an error to the invoking process.
Code Block | ||||
---|---|---|---|---|
| ||||
int main(int argc, char** argv) { try { Object object; // do useful work return 0; // object gets destroyed here } catch (...) { exit(EXIT_FAILURE); } } |
...
In the following code example, the function f()
claims to throw exception1
but actually throws exception2
. Consequently control flow is diverted to std::unexpected
, and the toplevel catch
clause may not be invoked. (It is not invoked on Linux with G++ 4.3).
Code Block | ||||
---|---|---|---|---|
| ||||
using namespace std; class exception1 : public exception {}; class exception2 : public exception {}; void f(void) throw( exception1) { // ... throw (exception2()); } int main() { try { f(); return 0; } catch (...) { cerr << "F called" << endl; } return EXIT_FAILURE; } |
...
The following code example declares the same exception it actually throws
Code Block | ||||
---|---|---|---|---|
| ||||
using namespace std; class exception1 : public exception {}; class exception2 : public exception {}; void f(void) throw( exception1) { // ... throw (exception1()); } int main() { try { f(); return 0; } catch (...) { cerr << "F called" << endl; } return EXIT_FAILURE; } |
...