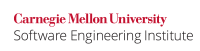
...
In this noncompliant code example, a std::vector
is used in place of a pointer and size pair. The function performs a range check to ensure that pos
does not exceed the upper bound of the array container but fails to check the lower bound for table
. Because pos
is declared as a (signed) int
, this parameter can assume a negative value, resulting in a write outside the bounds of the std::vector
object.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <vector> void insert_in_table(std::vector<int> &table, int pos, int value) { if (pos >= table_.size()) { // Handle error return; } table[pos] = value; } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <vector> void insert_in_table(std::vector<int> &table, std::size_t pos, int value) { if (pos >= table_.size()) { // Handle error return; } table[pos] = value; } |
...