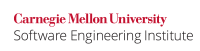
...
Class | Function | Iterators | References | Pointers | Notes |
---|---|---|---|---|---|
std::deque | |||||
insert() , emplace_front() , emplace_back() ,emplace() , push_front() , push_back() | X | X | An insertion in the middle of the deque invalidates all the iterators and references to elements of the deque. An insertion at either end of the deque invalidates all the iterators to the deque but has no effect on the validity of references to elements of the deque. ([deque.modifiers], paragraph 1) | ||
erase() , pop_back() , resize() | X | X | An erase operation that erases the last element of a deque invalidates only the past-the-end iterator and all iterators and references to the erased elements. An erase operation that erases the first element of a deque but not the last element invalidates only the erased elements. An erase operation that erases neither the first element nor the last element of a deque invalidates the past-the-end iterator and all iterators and references to all the elements of the deque. ([deque.modifiers], paragraph 4) | ||
clear() | X | X | X | Destroys all elements in the container. Invalidates all references, pointers, and iterators referring to the elements of the container and may invalidate the past-the-end iterator. ([sequence.reqmts], Table 100) | |
std::forward_list | |||||
erase_after() , pop_front() , resize() | X | X | erase_after shall invalidate only iterators and references to the erased elements. ([forwardlist.modifiers], paragraph 1) | ||
remove() , unique() | X | X | Invalidates only the iterators and references to the erased elements. ([forwardlist.ops], paragraph 12 & paragraph 16) | ||
clear() | X | X | X | Destroys all elements in the container. Invalidates all references, pointers, and iterators referring to the elements of the container and may invalidate the past-the-end iterator. ([sequence.reqmts], Table 100) | |
std::list | |||||
erase() , pop_front() , pop_back() , clear() , remove() , remove_if() , unique() | X | X | Invalidates only the iterators and references to the erased elements. ([list.modifiers], paragraph 3 and [list.ops], paragraph 15 & paragraph 19) | ||
clear() | X | X | X | Destroys all elements in the container. Invalidates all references, pointers, and iterators referring to the elements of the container and may invalidate the past-the-end iterator. ([sequence.reqmts], Table 100) | |
std::vector | |||||
reserve() | X | X | X | After | |
insert() , emplace_back() , emplace() , push_back() | X | X | Causes reallocation if the new size is greater than the old capacity. If no reallocation happens, all the iterators and references before the insertion point remain valid. ([vector.modifiers], paragraph 1). All iterators and references after the insertion point are invalidated. | ||
erase() , pop_back() , resize() | X | X | Invalidates iterators and references at or after the point of the erase. ([vector.modifiers], paragraph 3) | ||
clear() | X | X | X | Destroys all elements in the container. Invalidates all references, pointers, and iterators referring to the elements of the container and may invalidate the past-the-end iterator. ([sequence.reqmts], Table 100) | |
std::set , std::multiset , std::map , std::multimap | |||||
erase() , clear() | X | X | Only invalidates iterators and references to the erased elements. ([associative.reqmts], paragraph 9) | ||
| |||||
erase() , clear() | X | X | Only invalidates Invalidates only iterators and references to the erased elements. ([unord.req], paragraph 14) | ||
insert() , emplace() | X | The | |||
rehash() , reserve() | X | Rehashing invalidates iterators, changes ordering between elements, and changes which buckets the elements appear in but does not invalidate pointers or references to elements. ([unord.req], paragraph 9) | |||
std::valarray | resize() | X | X | Resizing invalidates all pointers and references to elements in the array. ([valarray.members], paragraph 12) |
...
In this compliant solution, pos
is assigned a valid iterator on each insertion, removing the undefined behavior:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <deque> void f(const double *items, std::size_t count) { std::deque<double> d; auto pos = d.begin(); for (std::size_t i = 0; i < count; ++i, ++pos) { pos = d.insert(pos, items[i] + 41.0); } } |
...
In this noncompliant code example, data
is invalidated after the call to replace()
, and so its use in g()
is undefined behavior:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <iostream> #include <string> extern void g(const char *); void f(std::string &example_string) { const char *data = example_string.data(); // ... example_string.replace(0, 2, "bb"); // ... g(data); } |
...
[ISO/IEC 14882-2014] | 23, "Containers Library" |
[Meyers 01] | Item 43: , Prefer algorithm calls to hand-written loops |
[Sutter 04] | Item 84: , Prefer algorithm calls to handwritten loops |
[Kalev 99] | ANSI/ISO C++ Professional Programmer's Handbook |
...