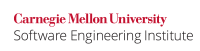
...
The solution is to ensure that the PRNG is always properly seeded with an initial seed value that will not be predictable or controllable by an attacker. A properly seeded PRNG will generate a different sequence of random numbers each time it is run.
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <random>
#include <iostream>
void f() {
std::mt19937 engine;
for (int i = 0; i < 10; ++i) {
std::cout << engine() << ", ";
}
}
output:
1st run: 3499211612, 581869302, 3890346734, 3586334585, 545404204, 4161255391, 3922919429, 949333985, 2715962298, 1323567403,
2nd run: 3499211612, 581869302, 3890346734, 3586334585, 545404204, 4161255391, 3922919429, 949333985, 2715962298, 1323567403,
...
nth run: 3499211612, 581869302, 3890346734, 3586334585, 545404204, 4161255391, 3922919429, 949333985, 2715962298, 1323567403,
|
Noncompliant Code Example
In this noncompliant code example, the random number generation engine is seeded with the current time. This is an improvement over the previous noncompliant code example, but is still unsuitable when an attacker can control the time at which the seeding is executed. Predictable seed values can result in exploits when the subverted PRNG is used.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <ctime> #include <random> #include <iostream> void f() { std::time_t t; std::mt19937 engine(std::time(&t)); for (int i = 0; i < 10; ++i) { std::cout << engine() << ", "; } } |
Compliant Solution
This compliant solution uses std::random_device
to generate a random seed value to see the Mersenne Twister engine object. The values generated by std::random_device
are nondeterministic random numbers when possible, relying on random number generation devices, like /dev/random
. When such a device is not available, std::random_device
may employ a random number engine; however, the initial value generated should have sufficient randomness to serve as a seed value.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <random> #include <iostream> void f() { std::random_device dev; std::mt19937 engine(dev()); for (int i = 0; i < 10; ++i) { std::cout << engine() << ", "; } } output: 1st run: 3921124303, 1253168518, 1183339582, 197772533, 83186419, 2599073270, 3238222340, 101548389, 296330365, 3335314032, 2nd run: 2392369099, 2509898672, 2135685437, 3733236524, 883966369, 2529945396, 764222328, 138530885, 4209173263, 1693483251, 3rd run: 914243768, 2191798381, 2961426773, 3791073717, 2222867426, 1092675429, 2202201605, 850375565, 3622398137, 422940882, ... |
Risk Assessment
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MSC51-CPP | Medium | Likely | Low | P18 | L1 |
...
Tool | Version | Checker | Description |
---|---|---|---|
Related Vulnerabilities
Using a predictable seed value, such as the current time, result in numerous vulnerabilities, such as the one described by CVE-2008-1637.
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
...
CERT C Coding Standard | MSC32-C. Properly seed pseudorandom number generators |
MITRE CWE | CWE-327, Use of a Broken or Risky Cryptographic Algorithm |
Bibliography
[ISO/IEC 14882-2014] | 26.5, "Random Number Generation" |
[ISO/IEC 9899:2011] | 7.22.2, "Pseudo-random Sequence Generation Functions" |
...