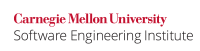
...
Guarantee | Description | Example |
---|---|---|
Strong | The strong exception safety guarantee is a property of an operation such that, in addition to satisfying the basic exception safety guarantee, if the operation terminates by raising an exception, it has no observable effects on program state. (See strong exception safety.) | |
Basic | The basic exception safety guarantee is a property of an operation such that, if the operation terminates by raising an exception, it preserves program state invariants and prevents resource leaks. (See basic exception safety.) | |
None | Code that provides neither the strong nor the basic exception safety guarantee is not exception safe. |
...
The following noncompliant code example shows a flawed implementation of the copy assignment operator of a dynamically sizable array class. The implicit invariants of the class are that the array
member is a valid (possibly null) pointer and that the nelems
member stores the number of elements in the array pointed to by array
.The copy assignment operator The code is flawed because it fails to provide any exception safety guarantee. The function deallocates array
and assigns the element counter, nelems
, before allocating a new block of memory for the copy. As a result, when if the new
expression expression throws an exception, the function will have modified the state of both member variables in a way that violates the implicit invariants of the class. Consequently, the such an object of the class is in an indeterminate state and any operation on it, including its destruction, results in undefined behavior.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <cstring> class IntArray { int *array; std::size_t nelems; public: // ... ~IntArray() { delete[] array; } IntArray& operator=(const IntArray &rhs) { if (this != &rhs) { delete[] array; array = nullptr; nelems = rhs.nelems; if (nelems) { array = new int[nelems]; std::memcpy(array, rhs.array, nelems * sizeof (*array)); } } return *this; } // ... }; |
...
In this compliant solution, the copy assignment operator provides the strong exception safety guarantee. The function takes care to allocate allocates new storage for the copy before changing the state of the object. Only after the allocation succeeds does the function proceed to change the state of the object. In addition, by copying the array to the newly allocated storage before deallocating the existing array, the function avoids the test for self-assignment, which improves the performance of the code in the common case.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <cstring> class IntArray { int *array; std::size_t nelems; public: // ... ~IntArray() { delete[] array; } IntArray& operator=(const IntArray &rhs) { int * consttmp = nullptr; if (rhs.nelems) { tmp = new int[rhs.nelems]; std::memcpy(tmp, rhs.array, nelems * sizeof (*array)); } delete[] array; array = tmp; nelems = rhs.nelems; return *this; } // ... }; |
...
Code that is not exception safe typically leads to resource leaks, causes the program to be left in an inconsistent or unexpected state, and ultimately results in undefined in undefined behavior at some point after the first exception is thrown.
...
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Parasoft C/C++test | 9.5 | BD-RES-LEAKS | |||||||
| 4075, 4076 |
Other Languages
TO DO
Bibliography
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
MITRE CWE | [CWE] | CWE-390, Detection of Error Condition Without ActionCWE-703, Failure to Handle Exceptional Conditions |
Bibliography
[ISO/IEC 14882-20032014] | Clause 15, "Exception Handling" | |
[ | MISRA 08]Rule 15-3-2 (Advisory)Stroustrup 01] | |
[Sutter 00] | Exceptional C++: 47 Engineering Puzzles, Programming Problems, and Solutions | |
[Sutter 01] | More Exceptional C++: 40 New Engineering Puzzles, Programming Problems, and Solutions |
...