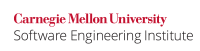
The C standard library facilities setjmp()
and longjmp()
can be used to simulate throwing and catching exceptions. However, these facilities bypass automatic resource management and can result in undefined behavior, commonly including resource leaks, and denial-of-service attacks.
...
Calling longjmp()
such that it would invoke a nontrivial destructor were the call replaced with a throw
expression results in undefined behavior, as demonstrated in this noncompliant code example.:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <csetjmp> #include <iostream> static jmp_buf env; struct Counter { static int Instances; Counter() { ++Instances; } ~Counter() { --Instances; } }; int Counter::Instances = 0; void f() { Counter c; std::cout << "f(): Instances: " << Counter::Instances << std::endl; std::longjmp(env, 1); } int main() { std::cout << "Before setjmp(): Instances: " << Counter::Instances << std::endl; if (setjmp(env) == 0) { f(); } else { std::cout << "From longjmp(): Instances: " << Counter::Instances << std::endl; } std::cout << "After longjmp(): Instances: " << Counter::Instances << std::endl; } |
...