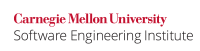
...
Code Block | ||||
---|---|---|---|---|
| ||||
struct alignas(32) Vector { char elems[32]; }; Vector *f() { Vector *pv = new Vector; return pv; } |
...
In this compliant solution, an overloaded operator new
function is defined to obtain appropriately aligned storage by calling the C11 function aligned_alloc()
. Programs that make use of the array form of the new expression must define the corresponding member array operator new[]
and operator delete[]
. The aligned_alloc()
function is not part of the C++ 98, C++ 11, or C++ 14 standards but may be provided by implementations of such standards as an extension. Programs targeting C++ implementations that do not provide the C11 aligned_alloc()
function must define the member operator new
to adjust the alignment of the storage obtained by the allocation function of their choice, either the default global operator new
or malloc()
.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <cstdlib> #include <new> struct alignas(32) Vector { char elems[32]; static void * operator new(size_t); static void operator delete(void *p) { free(p); } }; void *Vector::operator new(size_t nbytes) { nbytes) { if (void *p = std::aligned_alloc(alignof(Vector), nbytes)) { return p; } throw std::bad_alloc(); } static void operator delete(void *p) { free(p); } }; Vector *f() { Vector *pv = new Vector; return pv; } |
...