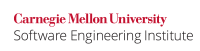
...
It is acceptable to define a deleted allocation or deallocation function without its corresponding free store function. For instance, it is a common practice to define a deleted non-placement allocation or deallocation function as a class member function when the class also defines a placement new
function. This prevents accidental allocation via calls to new
for that class type , or deallocation via calls to delete
on pointers to an object of that class type. It is acceptable to declare, but not define, a private allocation or deallocation function without its corresponding free store function for similar reasons. However, a definition must not be provided as that still allows access to the free store function within a class member function.
...
In this compliant solution, the corresponding deallocation function is also defined at global scope:.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <Windows.h> #include <new> class HeapAllocator { static HANDLE h; static bool init; public: static void *alloc(std::size_t size) noexcept(false) { if (!init) { h = ::HeapCreate(0, 0, 0); // Private, expandable heap. init = true; } if (h) { return ::HeapAlloc(h, 0, size); } throw std::bad_alloc(); } static void dealloc(void *ptr) noexcept { if (h) { (void)::HeapFree(h, 0, ptr); } } }; HANDLE HeapAllocator::h = nullptr; bool HeapAllocator::init = false; void *operator new(std::size_t size) noexcept(false) { return HeapAllocator::alloc(size); } void operator delete(void *ptr) noexcept { return HeapAllocator::dealloc(ptr); } |
...
In this compliant solution, the corresponding operator delete()
is overloaded at the same class scope:.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <new> extern "C++" void update_bookkeeping(void *allocated_ptr, std::size_t size, bool alloc); struct S { void *operator new(std::size_t size) noexcept(false) { void *ptr = ::operator new(size); update_bookkeeping(ptr, size, true); return ptr; } void operator delete(void *ptr, std::size_t size) noexcept { ::operator delete(ptr); update_bookkeeping(ptr, size, false); } }; |
...