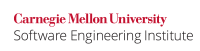
...
Code Block | ||||
---|---|---|---|---|
| ||||
Widget w( /* constructor arguments */);
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
Widget w = Widget( /* constructor arguments */);
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
Widget w = /* constructor argument */;
|
Besides being inefficient, this last syntax violates OOP32OOP09-CPP. Ensure that single-argument constructors are marked "explicit".
...
Code Block | ||||
---|---|---|---|---|
| ||||
class Widget {
public:
explicit Widget() {cerr << "constructed" << endl;}
};
int main() {
Widget w();
return 0;
}
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
class Widget {
public:
explicit Widget() {cerr << "constructed" << endl;}
};
int main() {
Widget w;
return 0;
}
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
class Widget {
public:
explicit Widget(int in) : i(in) {cerr << "widget constructed" << endl;}
private:
int i;
};
class Gadget {
public:
explicit Gadget(Widget wid) : w(wid) {cerr << "gadget constructed" << endl;}
private:
Widget w;
};
int main() {
int i = 3;
Gadget g(Widget(i));
cout << i << endl;
return 0;
}
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
Gadget g(Widget i);
|
As a result, this program compiles cleanly and prints only 3
as output, because no Gadget
or Widget
is constructed.
...
Code Block | ||||
---|---|---|---|---|
| ||||
int main() {
int i = 3;
Widget w(i);
Gadget g(w);
cout << i << endl;
return 0;
}
|
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
OOP31-CPP | low | likely | low | P9 | L2 |
Automated Detection
Tool | Version | Checker | Description | ||||||
| 2510 |
Bibliography
- [Meyers 01] Item 6: Be alert for C++'s most vexing parse.
...