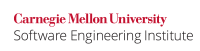
...
Code Block | ||||
---|---|---|---|---|
| ||||
enum { TABLESIZE = 100 };
int *table = NULL;
int insert_in_table(int pos, int value){
if (!table) {
table = new int[TABLESIZE];
}
if (pos >= TABLESIZE) {
return -1;
}
table[pos] = value;
return 0;
}
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
enum { TABLESIZE = 100 };
int *table = NULL;
int insert_in_table(size_t pos, int value){
if (!table) {
table = new int[TABLESIZE];
}
if (pos >= TABLESIZE) {
return -1;
}
table[pos] = value;
return 0;
}
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
template <typename T>
void clear(T array[], size_t n) {
for (size_t i = 0; i < n; ++i) {
array[i] = 0;
}
}
template <typename T, size_t n>
inline void clear(T (&array)[n]) {
clear(array, n);
}
int int_array[12];
clear(int_array); // deduce T is int, and that n is 12
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
vector<int> table;
int insert_in_table(int pos, int value){
if (pos >= table.size()) {
return -1;
}
table[pos] = value;
return 0;
}
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
vector<int> table;
int insert_in_table(size_t pos, int value){
if (pos >= table.size()) {
return -1;
}
table[pos] = value;
return 0;
}
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
vector<int> table;
int insert_in_table(size_t pos, int value){
table.at(pos) = value;
return 0;
}
|
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
ARR30-CPP | high | likely | high | P9 | L2 |
Automated Detection
...
The LDRA tool suite Version 7.6.0 can detect violations of this rule.
...
Tool | Version | Checker | Description |
---|---|---|---|
Coverity | 5.0 |
...
NEGATIVE_RETURNS | Implemented | ||
LDRA | 7.6 | Implemented | |
Klockwork |
8.0.4.16 |
...
ABR ABV.TAINTED |
...
SV.TAINTED.INDEX_ACCESS |
...
Implemented | |
Compass/ROSE |
...
Implemented |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
...