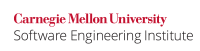
When an exception is thrown, control is transferred to the nearest handler with a type that matches the type of the exception thrown. If no matching handler is directly found within the handlers for a try block in which the exception is thrown, the search for a matching handler continues to dynamically search for handlers in the surrounding try blocks of the same thread. The C++ Standard, [except.handle], paragraph 9 [ISO/IEC 14882-2014], states the following:
If no matching handler is found, the function
std::terminate()
is called; whether or not the stack is unwound before this call tostd::terminate()
is implementation-defined.
...
In this compliant solution, the main entry point handles all exceptions, which ensures that the stack is unwound up to the main()
function and allows for graceful management of external resources:.
Code Block | ||||
---|---|---|---|---|
| ||||
void throwing_func() noexcept(false); void f() { throwing_func(); } int main() { try { f(); } catch (...) { // Handle error } } |
...
In this compliant solution, the thread_start()
handles all exceptions and does not rethrow, allowing the thread to terminate normally:.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <thread> void throwing_func() noexcept(false); void thread_start(void) { try { throwing_func(); } catch (...) { // Handle error } } void f() { std::thread t(thread_start); t.join(); } |
...