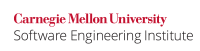
...
Code Block | ||
---|---|---|
| ||
public class DivideException { public static void main(String[] args) { try { division(200, 5); division(200, 0); // Divide by zero } catch (Exception e) { System.out.println("Divide by zero exception : " + e.getMessage()); } } public static void division(int totalSum, int totalNumber) throws ArithmeticException, IOException { int average = totalSum / totalNumber; // Additional operations that may throw IOException... System.out.println("Average: " + average); } } |
...
Code Block | ||
---|---|---|
| ||
try { division(200, 5); division(200, 0); // Divide by zero } catch (ArithmeticException ae) { throw new DivideByZeroException(); } catch (Exception e) { System.out.println("Exception occurred :" + e.getMessage()); } |
...
Code Block | ||
---|---|---|
| ||
import java.io.IOException; public class DivideException { public static void main(String[] args) { try { division(200,5); division(200,0); // Divide by zero } catch (ArithmeticException ae) { throw new DivideByZeroException(); // DivideByZeroException extends Exception so is checked } catch (IOException ie) { System.out.println("I/O Exception occurred :" + ie.getMessage()); } } public static void division(int totalSum, int totalNumber) throws ArithmeticException, IOException { int average = totalSum / totalNumber; // Additional operations that may throw IOException... System.out.println("Average: "+ average); } } |
...
EXC14-EX0: A catch block may catch all exceptions in order to process them before re-throwing them. For example:
...
In such cases, a catch block should catch Throwable
rather than Exception
or RuntimeException
.
Wiki Markup |
---|
*EXC14-EX2EX1*: Task processing threads such as worker threads in a thread pool or the swing event dispatch thread are permitted to catch {{RuntimeException}} when they call untrusted code through an abstraction such as {{Runnable}} \[[Goetz 2006 pg 161|AA. Bibliography#Goetz 06]\]. |
EXC14-EX3EX2: Systems that require substantial fault tolerance or graceful degradation are permitted to catch and log general exceptions such as Throwable
at appropriate levels of abstraction. Permitted examples includeFor example:
- A realtime control system that catches and logs all exceptions at the outermost layer, followed by warm-starting the system so that realtime control can continue. Such approaches are clearly justified when program termination would have safety-critical or mission-critical consequences.
- A system that catches all exceptions that propagate out of each major subsystem, logs the exceptions for later debugging, and subsequently shuts down the failing subsystem (perhaps replacing it with a much simpler, limited-functionality version) while continuing other services.
...