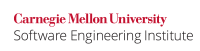
...
A reviewer may now recognize that the operation should also be checked for overflow. This might not have been apparent in the original, noncompliant code example. For more information, see INT00-J. Ensure that integer operations do not result in overflow.
Noncompliant Code Example (Logical Right Shift)
In this noncompliant code example, the programmer prematurely optimizes attempts to optimize code by replacing a division with a right shift.
Code Block | ||
---|---|---|
| ||
int x = -50;
x = x + 2;
x >>>= 2;
|
The >>>=
operator is a logical right shift; it fills the leftmost bits with zeroes, regardless of the number's original sign. After execution of this code sequence, x
contains a large positive number (specifically, 0x3FFFFFF3
). Using logical right shift for division produces an incorrect result when the dividend (x
in this example) contains a negative value.
Noncompliant Code Example (Arithmetic Right Shift)
In this noncompliant code example, the programmer attempts to correct the previous example by using an arithmetic right shift (the >>=
operator) can replace division, as shown in the following example:
Code Block | ||
---|---|---|
| ||
int x = -50;
x = x + 2;
x >>= 2;
|
However, the result is less maintainable than making the division explicit. Refer to guideline NUM04-J. Use shift operators correctly for examples of sign extension with respect to the shift operatorsAfter execution of this code sequence, x
contains the value -13
rather than the expected -12
. Arithmetic right shift truncates its results towards negative infinity; integer division truncates towards zero.
Compliant Solution (Right Shift)
In this compliant solution, the right shift is correctly replaced by division.
Code Block | ||
---|---|---|
| ||
int x = -50; x += 2 x /= 4; |
Noncompliant Code Example
...
NUM02-EX0: Bitwise operations may be used to construct constant expressions. As a matter of style, we recommend replacing such constant expressions with the equivalent hexadecimal constants; 0x1FFFF in this case.
Code Block | ||
---|---|---|
| ||
int limit = 1 << 17 - 1; // 2^17 - 1 = 131071 |
NUM02-EX1: Data that is normally treated arithmetically may be treated with bitwise operations for the purpose of serialization or deserialization. This is often required for reading or writing the data from a file or network socket, . It may also be used when reading or writing the data from a tightly packed data structure of bytes.
...