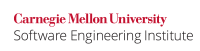
...
Wiki Markup |
---|
Autoboxing automatically wraps a value of a primitive type with the corresponding wrapper object. The [§5§5.1.7 "Boxing Conversion,"|http://java.sun.com/docs/books/jls/third_edition/html/conversions.html#5.1.7] of the _Java Language Specification_ \[[JLS 2005|AA. Bibliography#JLS 05]\] explains which primitive values are memoized during autoboxing: |
...
Less memory-limited implementations could, for example, cache all characters and shorts, as well as integers and longs in the range of -32K ┠— +32K.
Code that depends on implementation-defined behavior is not portable.
...
Code Block | ||
---|---|---|
| ||
public class Wrapper {
public static void main(String[] args) {
// Create an array list of integers, where each element
// is greater than 127
ArrayList<Integer> list1 = new ArrayList<Integer>();
for (int i = 0; i < 10; i++) {
list1.add(i + 1000);
}
// Create another array list of integers, where each element
// has the same value as the first list
ArrayList<Integer> list2 = new ArrayList<Integer>();
for (int i = 0; i < 10; i++) {
list2.add(i + 1000);
}
// Count matching values.
int counter = 0;
for (int i = 0; i < 10; i++) {
if (list1.get(i) == list2.get(i)) { // uses '=='
counter++;
}
}
// print the counter: 0 in this example
System.out.println(counter);
}
}
|
If the particular JVM running this code memoized integer values from -32,768 to 32,767, all of the int
values in the example would have been autoboxed to singleton Integer
objects, and the example code would have operated as expected. Using reference equality instead of object equality requires that all values encountered fall within the interval of values memoized by the JVM. The Java Language Specification does not specify this interval; it only provides a minimum range. Consequently, successful prediction of this program's behavior would require implementation-specific details of the JVM.
...
Wiki Markup |
---|
\[[Bloch 2009|AA. Bibliography#Bloch 09]\] 4. "Searching for the One" \[[JLS 2005|AA. Bibliography#JLS 05]\] [§5§5.1.7|http://java.sun.com/docs/books/jls/third_edition/html/conversions.html#5.1.7], "Boxing Conversion" \[[Pugh 2009|AA. Bibliography#Pugh 09]\] Using == to compare objects rather than .equals |
...
EXP02-J. Use the two-argument Arrays.equals() method to compare the contents of arrays 02. Expressions (EXP) EXP04-J. Do not perform assignments in conditional statements