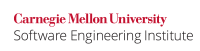
...
Code Block | ||
---|---|---|
| ||
switch(x) { case 0: foo(); break; case 1: bar(); break; } const char* f(Color c) { switch (c) { case Red: return "Red"; case Green: return "Green"; case Blue: return "Blue"; default: return "Unknown color"; /* necessary */ } } |
Note that adding a default case to a switch statement, even when all possible switch labels are specified, is exception (MSC07-EX1) to recommendation MSC07-C. Detect and remove dead code.
An alternative compliant solution to the noncompliant code example above is to provide a return
statement after the switch
statement. Note, however, that this solution may not be appropriate in all situations.
Code Block | ||
---|---|---|
| ||
typedef enum { Red, Green, Blue } Color; const char* f(Color c) { switch (c) { case Red: return "Red"; case Green: return "Green"; case Blue: return "Blue"; } return "Unknown color"; /* necessary */ } |
...
This practice has been a subject of debate for some time, but a clear direction has emerged.
Originally, the consensus among those writing best practices was simply that each switch
statement should have a default
label. Eventually, emerging compilers and static analysis tools could verify that a switch
on an enum
type contained a case
label for each enumeration value, but only if no default
label existed. This led to a shift toward purposely leaving out the default
label to allow static analysis. However, the resulting code was then vulnerable to enum
variables being assigned int
values outside the set of enum
values.
These two practices have now been merged. A switch
on an enum
type should now contain a case
label for each enum
value, but should also contain a default
label for safety. This is not more difficult to analyze statically.
Existing implementations are in transition, with some not yet analyzing switch
statements with default
labels. Developers must take extra care to check their own switch
statements until the new practice becomes universal.
Noncompliant Code Example (Zune 30)
...
Tool
...
Version
...
Checker
...
Description
...
Section |
---|
...
...
...
Section |
---|
GCC |
...
...
Section |
---|
can detect some violations of this recommendation when the |
...
Section |
---|
Compass/ROSE |
...
...
...
Section | |||||
---|---|---|---|---|---|
can detect some violations of this recommendation. In particular, it flags switch statements that do not have a default clause. ROSE should also detect "fake switches," as well (that is, a chain of
|
...
Section |
---|
...
Section |
---|
LA_UNUSED |
...
...
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
CERT C++ Secure Coding Standard: MSC01-CPP. Strive for logical completeness
This rule appears in the C Secure Coding Standard as MSC01-C. Strive for logical completeness.
ISO/IEC TR 24772 "CLL Switch statements and static analysis"
...