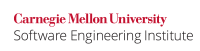
...
As a result, code that depends upon the remainder operation to always return a positive result is erroneous.
Noncompliant Code Example
This noncompliant code example uses the integer hashKey
as an index into the hash
array. A negative hash key produces a negative result from the remainder operator causing the lookup()
method to throw java.lang.ArrayIndexOutOfBoundsException
.
Code Block | ||
---|---|---|
| ||
private int SIZE = 16; public int[] hash = new int[SIZE]; public int lookup(int hashKey) { return hash[hashKey % SIZE]; } |
Compliant Solution
This compliant solution calls the imod()
method that always returns a positive remainder.
Code Block | ||
---|---|---|
| ||
// method imod() gives non-negative result private int SIZE = 16; public int[] hash = new int[SIZE]; private int imod(int i, int j) { int temp = i % j; return (temp < 0) ? -temp : temp; // unary - will succeed without overflow // because temp cannot be Integer.MIN_VALUE } public int lookup(int hashKey) { return hash[imod(hashKey, SIZE)]; } |
Risk Assessment
Incorrectly assuming a positive remainder from a remainder operation can result in erroneous code.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
NUM00 NUM16-J | low | unlikely | high | P1 | L3 |
Automated Detection
Automated detection of uses of the %
operator is straightforward. Sound determination of whether those uses correctly reflect the intent of the programmer is infeasible in the general case. Heuristic warnings could be useful.
Related Guidelines
C Secure Coding Standard | "INT10-C. Do not assume a positive remainder when using the % operator" |
C++ Secure Coding Standard | "INT10-CPP. Do not assume a positive remainder when using the % operator" |
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="667b84ebc3b882ce-dbf3b59b-484343e5-a8e68aeb-61a54ed74753cd4c2937ef08"><ac:plain-text-body><![CDATA[ | [[JLS 2005 | AA. Bibliography#JLS 05]] | [§15.17.3, "Remainder Operators" | http://java.sun.com/docs/books/jls/third_edition/html/expressions.html#15.17.3] | ]]></ac:plain-text-body></ac:structured-macro> |
...