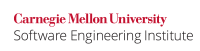
...
Noncompliant Code Example
This noncompliant code example corrupts the data when string
contains characters that are not representable in the specified charset
.
Code Block | ||
---|---|---|
| ||
// Corrupts data on errors public static byte[] toCodePage(String charset, String string) throws UnsupportedEncodingException { return string.getBytes(charset); } // Fails to detect corrupt data public static String fromCodePage(String charset, byte[] bytes) throws UnsupportedEncodingException { return new String(bytes, charset); } |
Compliant Solution
The java.nio.charset.CharsetEncoder
class can transform a sequence of 16-bit Unicode characters into a sequence of bytes in a specific charset
, while the java.nio.charset.CharacterDecoder
class can reverse the procedure [API 2006].
...
Code Block | ||
---|---|---|
| ||
public static byte[] toCodePage(String charset, String string) throws IOException { Charset cs = Charset.forName(charset); CharsetEncoder coder = cs.newEncoder(); ByteBuffer bytebuf = coder.encode(CharBuffer.wrap(string)); byte[] bytes = new byte[bytebuf.limit()]; bytebuf.get(bytes); return bytes; } |
Noncompliant Code Example
This noncompliant code example attempts to append a string to a text file in the specified encoding. This is erroneous because the String
may contain unrepresentable characters.
Code Block | ||
---|---|---|
| ||
// Corrupts data on errors public static void toFile(String charset, String filename, String string) throws IOException { FileOutputStream stream = new FileOutputStream(filename, true); OutputStreamWriter writer = new OutputStreamWriter(stream, charset); writer.write(string, 0, string.length()); writer.close(); } |
Compliant Solution
This compliant solution uses the CharsetEncoder
class to perform the required function.
...
Use the FileInputStream
and InputStreamReader
objects to read back the data from the file. InputStreamReader
accepts a optional CharsetDecoder
argument, which must be the same as that previously used for writing to the file.
Exceptions
STR03-EX0: Binary data that is expected to be a valid string may be read and converted to a string. How to perform this operation securely is explained in rule STR04-J. Use compatible character encodings when communicating string data between processes.
Risk Assessment
Attempting to read a byte array containing binary data as if it were character data can produce erroneous results.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
STR03-J | low | unlikely | medium | P2 | L3 |
Related Guidelines
Bibliography