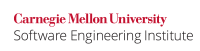
...
Code Block | ||
---|---|---|
| ||
// class Helper remains unchanged
final class RequestHandler {
private final Helper helper = new Helper();
private final ServerSocket server;
private final ExecutorService exec;
private RequestHandler(int port, int poolSize) throws IOException {
server = new ServerSocket(port);
exec = Executors.newFixedThreadPool(poolSize);
}
public static RequestHandler newInstance(int poolSize) throws IOException {
return new RequestHandler(0, poolSize);
}
public void handleRequest() {
Future<?> future = exec.submit(new Runnable() {
@Override public void run() {
try {
helper.handle(server.accept());
} catch (IOException e) {
// Forward to handler
}
}
});
}
// ... other methods such as shutting down the thread pool and task cancellation ...
}
|
...
The ExecutorService
interface used in this compliant solution derives from the java.util.concurrent.Executor
interface. The ExecutorService.submit()
method allows callers to obtain a Future<V>
object. This object encapuslates encapsulates the as-yet-unknown result of an asynchronous computation and enables callers to perform additional functions such as task cancellation.
Wiki Markup |
---|
The choice of the unbounded {{newFixedThreadPool}} is not always optimal. Refer to the Java API documentation for choosing between the following to meet specific design requirements \[[API 2006|AA. Java References#API 06]\]:
*{{ |
newFixedThreadPool()
...
newCachedThreadPool()
...
newSingleThreadExecutor()
...
newScheduledThreadPool()
...
Risk Assessment
Using simplistic concurrency primitives to process an unbounded number of requests may result in severe performance degradation, deadlock, or system resource exhaustion and denial of service.
...