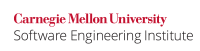
The enhanced for
statement introduced in Java 1.5, commonly referred to as the for-each idiom, is primarily used for iterating over collections of objects. While similar to the for
statement, this idiom cannot be used to assign values to the loop variableassignments to the loop variable do not modify the collection of objects over which the loop iterates. Assignments to the loop variable may not have the effect intended by the developer and should be avoided.
In more detail, according to the JLS an enhanced for
statement of the form:
Code Block |
---|
for (ObjType obj : someIterableItem) {
...
}
|
is equivalent to a standard for
loop of the form:
Code Block |
---|
for (Iterator myIterator = someIterableItem.iterator(); iterator.hasNext();) {
ObjType obj = myIterator.next();
...
}
|
Therefore, an assignment to the loop variable is equivalent to modifying a variable local to the loop body whose initial value is the collection item referred to by the loop iterator. While this modification is not necessarily erroneous, it may obscure the loop functionality or indicate a misunderstanding of the underlying implementation of the enhanced for
statement.
It is recommended that all enhanced for
statement loop variables be declared final
. The final
declaration will cause the Java compiler to flag and reject any assignments made to the loop variable in the loop body.
Noncompliant Code Example
This noncompliant example attempts to initialize a Character
array using an enhanced for
loop. However, because assignments to the loop variable cannot be assigned toto not modify the collection or array over which the loop is iterating, the array is not suitably initialized.
Code Block | ||
---|---|---|
| ||
Character[] array = new Character[10]; for(Character c: array) c = 'x'; // initialization attempt for(int i=0;i<array.length;i++) System.out.print(array[i]); // prints 10 "null"s |
Note that if c
was declared final
in the noncompliant code example the Java compiler would issue a compilation error regarding the "c = 'x';
statement.
Compliant Solution
This compliant solution correctly initializes the array using a for loop.
Code Block | ||
---|---|---|
| ||
Character[] array = new Character[10]; for(int i=0;i<array.length;i++) array[i] = 'x'; for(int i=0;i<array.length;i++final Character c: array) System.out.print(array[i]c); // prints 10 "x"s |
Risk Assessment
...