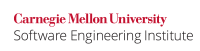
...
This compliant solution stops the thread by using a volatile
flag. An accessor method shutdown()
is used to set the flag to true
. The thread's run()
method polls the done
flag, and shuts down terminates when it becomes true
.
Code Block | ||
---|---|---|
| ||
public final class Container implements Runnable { private final Vector<Integer> vector = new Vector<Integer>(1000); private volatile boolean done = false; public Vector<Integer> getVector() { return vector; } public void shutdown() { done = true; } public synchronized void run() { Random number = new Random(123L); int i = vector.capacity(); while (!done && i > 0) { vector.add(number.nextInt(100)); i--; } } public static void main(String[] args) throws InterruptedException { Container container = new Container(); Thread thread = new Thread(container); thread.start(); Thread.sleep(5000); container.shutdown(); } } |
...
This method interrupts the current thread, however, it only stops the thread because the code polls the interrupted flag using the method Thread.interrupted()
, and shuts down when it is interrupted.
Upon receiving the interruption, the interrupted status of the thread is cleared and an InterruptedException
is thrown. No guarantees are provided by the JVM on when the interruption will be detected by blocking methods such as Thread.sleep()
and Object.wait()
. A thread may use interruption for performing tasks other than cancellation and shutdown. Consequently, a thread should not be interrupted unless its interruption policy is known in advance. Failure to follow this advice can result in the corruption of mutable shared state.
...