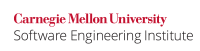
Thread-safety A consistent locking policy guarantees that no two threads can simultaneously access or modify some shared data. However, if two or more operations need to be performed as a single atomic operation, it becomes necessary to add additional locking to enforce atomicity. It In the absence of such a policy, it is possible for two threads to read some shared value, independently perform operations on it and induce a race condition while storing the final resultcondition while storing the final result. If two or more operations need to be performed as a single atomic operation, it is necessary to implement a consistent locking policy by either using synchronization or the java.util.concurrent
utilities.
In presence of an invariant involving two objects, it is tempting to believe that if operations on the two objects are individually atomic, no additional locking is required; however this is not the case. LikewiseSimilarly, programmers sometimes incorrectly assume that using a thread-safe Collection
does not require explicit synchronization to preserve an invariant involving the collection's elements. A thread-safe class can only guarantee atomicity of its individual methods. A grouping of calls to such methods requires additional synchronization.
For exampleinstance, consider a scenario where the standard thread-safe API does not provide a method to both find a particular person's record in a Hashtable
and also update the corresponding payroll information. In such cases, a custom atomic method must be designed and used. This guideline shows why discusses the rationale behind using such a method is necessary and suggests techniques for incorporating it using a custom APIand provides the necessary implementation advice.
This guideline applies to all Collection
classes including the thread-safe Hashtable
class. Enumerations of objects of a Collection
and iterators also require explicit synchronization on the Collection
object (client-side locking) or any single internal private lock object.
...
An AtomicReference
is an object reference that can be updated atomically. Operations that use these two atomic references independently, are guaranteed to be atomic, however, if an operation involves using both together, thread-safety issues arise. In this noncompliant code example, one thread may call update()
while a second thread may call add()
. This might cause the add()
operation to add the new value of first
to the old value of second
, yielding an erroneous result.
...
This compliant solution declares the update()
and add()
methods as synchronized
to guarantee atomicity.
Code Block | ||
---|---|---|
| ||
final class AtomicAdder {
// ...
public synchronized void update(BigInteger f, BigInteger s){
first.set(f);
second.set(s);
}
public synchronized BigInteger add() {
return first.get().add(second.get());
}
}
|
...
This noncompliant code example comprises an is comprised of a java.util.ArrayList<E>
collection which is not thread-safe by default. However, most most classes that are not thread-unsafe classes safe have a synchronized thread-safe version, for example, Collections.synchronizedList
is a good substitute for ArrayList
and Collections.synchronizedMap
is a good alternative to HashMap
. The atomicity pitfall described in the subsequent paragraph, remains to be addressed even when the particular Collection
offers thread-safety guarantees.
Code Block | ||
---|---|---|
| ||
final class IPHolder { private final List<InetAddress> ips = Collections.synchronizedList(new ArrayList<InetAddress>()); public void addIPAddress(InetAddress iaaddress) { // Validate iaaddress ips.add(iaaddress); } public void addAndPrintIP() throws UnknownHostException { addIPAddress(InetAddress.getLocalHost()); InetAddress[] ia = (InetAddress[]) ips.toArray(new InetAddress[0]); System.out.println("Number of IPs: " + ia.length); } } |
...
Code Block | ||
---|---|---|
| ||
final class IPHolder { private final List<InetAddress> ips = Collections.synchronizedList(new ArrayList<InetAddress>()); public void addIPAddress(InetAddress iaaddress) { synchronized (ips) { // Validate ips.add(iaaddress); } } public void addAndPrintIP() throws UnknownHostException { synchronized (ips) { addIPAddress(InetAddress.getLocalHost()); InetAddress[] ia = (InetAddress[]) ips.toArray(new InetAddress[0]); System.out.println("Number of IPs: " + ia.length); } } } |
...
Wiki Markup |
---|
Although expensive in terms of performance, the {{CopyOnWriteArrayList}} and {{CopyOnWriteArraySet}} classes are sometimes used to create copies of the core {{Collection}} so that iterators do not fail with a runtime exception when some data in the {{Collection}} is modified. However, any updates to the {{Collection}} are not immediately visible to other threads. Consequently, the use of these classes is limited to boosting performance in code where the writes are fewer (or non-existent) as compared to the reads \[[JavaThreads 04|AA. Java References#JavaThreads 04]\]. In most other cases they must be avoided (see [MSC13-J. Do not modify the underlying collection when an iteration is in progress] for details on using these classes). |
...
Wiki Markup |
---|
This noncompliant code example defines a class {{KeyedCounter}} which is not thread-safe. Even though the {{HashMap}} is fieldwrapped isin synchronized {{Map}}, the overall increment operation is not atomic. \[[Lee 09|AA. Java References#Lee 09]\] |
...
Compliant Solution (synchronized blocks)
This To ensure atomicity, this compliant solution uses a private internal lock object to synchronize the method bodies of the increment()
and getCount()
methods, to ensure atomicity.
Code Block | ||
---|---|---|
| ||
public final class KeyedCounter { private final Map<String,Integer> map = new HashMap<String, Integer>(); private final Object lock = new Object(); public void increment(String key) { synchronized (lock) { Integer old = map.get(key); int value = (old == null) ? 1 : old.intValue() + 1; map.put(key, value); } } public Integer getCount(String key) { synchronized (lock) { return map.get(key); } } } |
Note that this This compliant solution does not use Collections.synchronizedMap()
. This is because locking on the (unsynchronized) map provides sufficient thread-safety for this application. The guideline CON02-J. Always synchronize on the appropriate object enlists certain objects that should not be used for synchronization purposes, including any object returned by Collections.synchronizedMap()
.
...
Wiki Markup |
---|
The previous compliant solution does not scale very well because a class with several {{synchronized}} methods can be a potential bottleneck as far as acquiring locks is concerned, and may further yield a deadlock or livelock. The class {{ConcurrentHashMap}} provides several utility methods to perform atomic operations and is often a good choice, as demonstrated in this compliant solution \[[Lee 09|AA. Java References#Lee 09]\]. |
...