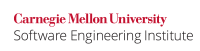
...
In this noncompliant code example, the PrintableIPAddressList
class extends the thread-safe IPAddressList
class. PrintableIPAddressList
locks on IpAddressListIPAddressList.ips
in the addAndPrintIPAddresses()
method. This is another example of client-side locking because a subclass is using an object owned and locked by its superclass.
Code Block | ||
---|---|---|
| ||
// This class may change its locking policy in the future, for example,
// if new non-atomic methods are added
class IPAddressList {
private final List<InetAddress> ips =
Collections.synchronizedList(new ArrayList<InetAddress>());
public List<InetAddress> getList() {
return ips; // No defensive copies required as package-private visibility
}
public void addIPAddress(InetAddress address) {
ips.add(address);
}
}
class PrintableIPAddressList extends IPAddressList {
public void addAndPrintIPAddresses(InetAddress address) {
synchronized(getList()) {
addIPAddress(address);
InetAddress[] ia = (InetAddress[]) getList().toArray(new InetAddress[0]);
// ...
}
}
}
|
...