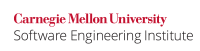
Wiki Markup |
---|
A boxing conversion converts the value of a primitive type to the corresponding value of the reference type, for instance, from {{int}} to the type {{Integer}} \[[JLS 2005|AA. Bibliography#JLS 05], Section 5.1.7|http://java.sun.com/docs/books/jls/third_edition/html/conversions.html#5.1.7] "Boxing Conversions"\]. ItThis can beis convenient in many cases where an object parameter is desired, such as with collection classes like {{Map}} and {{List}}. Another use case is to pass object references to methods, as opposed to primitive types that are always passed by value. The resulting wrapper types also help to reduce clutter in code. |
Noncompliant Code Example
...
Code Block | ||
---|---|---|
| ||
public class ShortSet { public static void main(String[] args) { HashSet<Short> s = new HashSet<Short>(); for(short i=0; i<100;i++) { s.add(i); s.remove(i - 1); } System.out.println(s.size()); } } |
Compliant Solution
Avoid mixing the different boxed integer types. If an arithmetic operation is expected to produce a primitive type which may get autoboxed to a wrong type, add explicit casts to the primitive type before allowing autoboxing to take overObjects removed from a collection should always share the type of the elements of the collection. Numeric promotion and autoboxing can produce unexpected object types. Ensure expected operation by using explicit casts to primitive types that parallel the intended boxed types.
Code Block | ||
---|---|---|
| ||
public class ShortSet { public static void main(String[] args) { HashSet<Short> s = new HashSet<Short>(); for(short i=0; i<100;i++) { s.add(i); s.remove((short)(i-1)); //cast to short } System.out.println(s.size()); } } |
...
Numeric promotion and autoboxing while removing elements from a Collection
, can make cause operations on the Collection
to fail silently.
Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
EXP11-J | low | probable | low | P6 | L2 |
Automated Detection
Detection of invocations of Collection.remove()
whose operand fails to match the type of the elements of the underlying collection is straightforward. It is possible, albeit unlikely, that some of these invocations may be intended. The remainder are heuristically likely to be in error.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
...