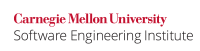
...
Compound operations on shared variables are also non-atomic. For more information, see guideline VNA02-J. Ensure that compound operations on shared variables are atomic.
CON04Guideline VNA04-J. Ensure that calls to chained methods are atomic describes a specialized case of this guideline.
...
Code Block | ||
---|---|---|
| ||
final class Adder { private final AtomicReference<BigInteger> first; private final AtomicReference<BigInteger> second; public Adder(BigInteger f, BigInteger s) { first = new AtomicReference<BigInteger>(f); second = new AtomicReference<BigInteger>(s); } public void update(BigInteger f, BigInteger s) { // Unsafe first.set(f); second.set(s); } public BigInteger add() { // Unsafe return first.get().add(second.get()); } } |
An AtomicReference
is an object reference that can be updated atomically. However, operations that combine more than one atomic reference are not non-atomic. In this noncompliant code example, one thread may call update()
while a second thread may call add()
. This might cause the add()
method to add the new value of first
to the old value of second
, yielding an erroneous result.
...
This compliant solution declares the update()
and add()
methods as synchronized to guarantee atomicity.
Code Block | ||
---|---|---|
| ||
final class Adder { // ... public synchronized void update(BigInteger f, BigInteger s){ first.set(f); second.set(s); } public synchronized BigInteger add() { return first.get().add(second.get()); } } |
...
This noncompliant code example uses a java.util.ArrayList<E>
collection, which is not thread-safe. However, the Collections.synchronizedList
is used as a synchronization wrapper for ArrayList
. An array, rather than an iterator, is used to iterate over Arraylist
to avoid a ConcurrentModificationException
.
...
Code Block | ||
---|---|---|
| ||
final class IPHolder { private final List<InetAddress> ips = Collections.synchronizedList(new ArrayList<InetAddress>()); public void addAndPrintIPAddresses(InetAddress address) { synchronized (ips) { ips.add(address); InetAddress[] addressCopy = (InetAddress[]) ips.toArray(new InetAddress[0]); } // Iterate through array addressCopy ... } } } |
Wiki Markup |
---|
This technique is also called client-side locking \[[Goetz 062006|AA. Java References#Goetz 06]\], because the class holds a lock on an object that might be accessible to other classes. Client-side locking is not always an appropriate strategy; see [CON34-J. Avoid client-side locking when using classes that do not commit to their locking strategy| guideline [LCK11-J. Avoid client-side locking when using classes that do not commit to their locking strategy] for more information. |
The addressCopy
array holds a copy of the IP addresses and can be safely operated upon outside the synchronized block. This code does not violate CON11guideline LCK04-J. Do not synchronize on a collection view if the backing collection is accessible because, because while it does synchronize on a collection view (the synchronizedList
), the backing collection is inaccessible , and therefore cannot be modified by any code.
...
Wiki Markup |
---|
This noncompliant code example defines a classthe {{KeyedCounter}} class that is not thread-safe. Although the {{HashMap}} is wrapped in a {{synchronizedMap}}, the overall increment operation is not atomic \[[Lee 092009|AA. Java References#Lee 09]\]. |
Code Block | ||
---|---|---|
| ||
final class KeyedCounter { private final Map<String, Integer> map = Collections.synchronizedMap(new HashMap<String, Integer>()); public void increment(String key) { Integer old = map.get(key); int oldValue = (old == null) ? 0 : old.intValue(); if (oldValue == Integer.MAX_VALUE) { throw new ArithmeticException("Out of range"); } map.put( key, oldValue + 1); } public Integer getCount(String key) { return map.get(key); } } |
Compliant Solution (
...
Synchronization)
To ensure atomicity, this compliant solution uses an internal private lock object to synchronize the statements of the increment()
and getCount()
methods.
...
This compliant solution does not use Collections.synchronizedMap()
because locking on the unsynchronized map provides sufficient thread-safety for this application. CON11Guideline LCK04-J. Do not synchronize on a collection view if the backing collection is accessible provides more information about synchronizing on synchronizedMap
objects.To prevent overflow, the caller must ensure that the increment()
method is called no more than Integer.MAX_VALUE
times for any key. See INT00-J. Perform explicit range checking to ensure integer operations do not overflow for more information.
Compliant Solution (ConcurrentHashMap
)
The previous compliant solution is safe for multithreaded use, however, but it does not scale well because of excessive synchronization, which can lead to contention and deadlock.
Wiki Markup |
---|
The {{ConcurrentHashMap}} class used in this compliant solution provides several utility methods for performing atomic operations and is often a good choice for algorithms that must scale \[[Lee 092009|AA. Java References#Lee 09]\]. |
...
Wiki Markup |
---|
According to Section 5.2.1., "ConcurrentHashMap" of the work of Goetz and colleagues \[[Goetz 062006|AA. Java References#Goetz 06]\]: |
...
Note that methods such as ConcurrentHashMap.size()
and ConcurrentHashMap.isEmpty()
are allowed to return an approximate result for performance reasons. Code should not rely on these return values for deriving exact results.
...
Failing to ensure the atomicity of two or more operations that need to be performed as a single atomic operation can result in race conditions in multithreaded applications.
Rule Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
CON03 VNA03- J | low | probable | medium | P4 | L3 |
Automated Detection
...
References
Wiki Markup |
---|
\[[API 062006|AA. Java References#API 06]\] \[[JavaThreads 042004|AA. Java References#JavaThreads 04]\] Section 8.2, "Synchronization and Collection Classes" \[[Goetz 062006|AA. Java References#Goetz 06]\] Section 4.4.1, "Client-side Locking," Section 5.2.1, "ConcurrentHashMap" \[[Lee 092009|AA. Java References#Lee 09]\] "Map & Compound Operation" |
...