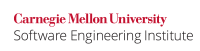
...
Code Block | ||
---|---|---|
| ||
public final class BookWrapper {
private final Book book;
private final Object lock = new Object();
BookWrapper(Book book) {
this.book = book;
}
public void issue(int days) {
synchronized(lock) {
book.issue();
}
}
public Calendar getDueDate() {
synchronized(lock) {
return book.getDueDate();
}
}
public void renew() {
synchronized(lock) {
if (book.getDueDate().after(Calendar.getInstance())) {
throw new IllegalStateException("Book overdue");
} else {
book.issue(14); // Issue book for 14 days
}
}
}
}
|
...