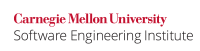
...
Code Block | ||
---|---|---|
| ||
public class TestWrapper2 { public static void main(String[] args) { Integer i1 = 100; Integer i2 = 100; Integer i3 = 1000; Integer i4 = 1000; System.out.println(i1.equals(i2)); System.out.println(i3.equals(i4)); } } |
...
In this noncompliant code example, it is desired to count the integers of arrays list1
and list2
. As class Integer
only caches integers from -127 to 128, when an int
value is beyond this range, it is autoboxed into the corresponding wrapper type. The ==
operator returns false
when these distinct wrapper objects are compared. As a result, the output of this example is 0.
Code Block | ||
---|---|---|
| ||
import java.util.ArrayList;
public class Wrapper {
public static void main(String[] args) {
// Create an array list of integers, where each element
// is greater than 127
ArrayList<Integer> list1 = new ArrayList<Integer>();
for(int i=0;i<10;i++)
list1.add(i+1000);
// Create another array list of integers, where each element
// is the same as the first list
ArrayList<Integer> list2 = new ArrayList<Integer>();
for(int i=0;i<10;i++)
list2.add(i+1000);
int counter = 0;
for(int i=0;i<10;i++)
if(list1.get(i) == list2.get(i))
counter++;
// print the counter
System.out.println(counter);
}
}
|
...
Code Block | ||
---|---|---|
| ||
Boolean b1 = true; Boolean b2 = true; if(b1 == b2) { // always equal // ... } |
If references are to be compared use the equals()
method instead of relational operators.
Risk Assessment
Using the equal and not equal operators to compare boxed primitives can lead to erroneous comparisons.
...