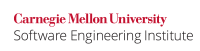
Holding a lock locks while performing operations that may block degrades performance, as threads that require the lock must wait for an unrelated block to finishtime consuming or blocking operations can severely degrade system performance and result in starvation. Furthermore, deadlock may result if too many interdependent threads block. Blocking operations includes include network I/O, invoking methods such as readLine()
and letting a thread defer itself indefinitely.
If the JVM is operating on a platform that uses a file system that operates over the network, then file I/O might also suffer the same performance hits as networked I/O. If such a platform is in use, then file I/O to files over the network should also not be performed avoided while a lock is held.
Noncompliant Code Example (intrinsic lock)
This noncompliant code example defines a utility method that accepts a time
parameter. Since the method is synchronized
, if the thread is suspended, other threads are unable to use the synchronized
methods of the class. In other words, the current object's monitor is not released.
Code Block | ||
---|---|---|
| ||
private synchronized void doSomething(long time) throws InterruptedException { // ... Thread.sleep(time); } |
Because the method is synchronized
, if the thread is suspended, other threads are unable to use the synchronized
methods of the class. In other words, the current object's monitor is not released.
Compliant Solution (intrinsic lock)
This compliant solution defines the synchronized
method with a timeout
parameter instead of the time
value. The use of the Object.wait()
method instead of Thread.sleep()
allows setting a time out for a period in which a notify signal may awaken the thread. The current object's monitor is immediately released upon entering the wait state. After the time out period has elapsed, the thread attempts to reacquire the current object's monitor and resumes execution when it succeeds.
Code Block | ||
---|---|---|
| ||
private synchronized void doSomething( long timeout) throws InterruptedException { while (<condition does not hold>) { wait(timeout); // Immediately releases lock on current monitor } } |
The current object's monitor is immediately released upon entering the wait state. After the time out period has elapsed, the thread attempts to reacquire the current object's monitor and resumes execution when it succeeds.
Wiki Markup |
---|
According to the Java API \[[API 06|AA. Java References#API 06]\], class {{Object}} documentation: |
...
CON20:EX1: A method that requires multiple locks may hold several locks while waiting for the remaining locks to be available. This constitutes a valid exception, but it though care must be done carefully taken to avoid deadlock. See CON12-J. Avoid deadlock by requesting and releasing locks in the same order for more information.
Risk Assessment
If a lock is held by a block that contains network transactional logic or thread deferrmentblocking or time consuming operations are performed within synchronized regions, temporary or permanent deadlocks deadlock may result.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
CON20-J | low | probable | high | P2 | L3 |
...