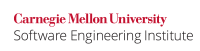
...
Consequently, either the &
or the &&
operator can be used when performing Boolean logic. However, there are times when the short-circuiting behavior is preferred and other times when the short-circuiting behavior causes subtle bugs.
Noncompliant Code Example (Improper &
)
Wiki Markup |
---|
This noncompliant code example, derived from Flanagan \[[Flanagan 2005|AA. Bibliography#Flanagan 05]\], has two variables, with no guarantees regarding their current values. The code must validate its data and then check whether {{array\[i\]}} is nonnegative. |
...
Wiki Markup |
---|
This code can fail as a result of the same errors it is attempting to prevent. When {{array}} is null or when {{i}} is not a valid index, the reference to {{array\[i\]}} will cause a {{NullPointerException}} or an {{ArrayIndexOutOfBoundsException}} to be thrown. This happens because the {{&}} operator fails to prevent evaluation of its right operand, even when evaluation of its left operand proves that the right operand is invalid. |
Compliant Solution (Use &&
)
This compliant solution mitigates the problem by using &&
, which causes the conditional expression to terminate immediately if any of the conditions fail, thereby preventing a potentially-invalid evaluation.
Code Block | ||
---|---|---|
| ||
int array[]; // may be null int i; // may be a valid index for array if (array != null && i >= 0 & i < array.length && array[i] >= 0) { // handle array } else { // handle error } |
Compliant Solution (Nested If Statements)
This compliant solution uses multiple if statements to achieve the proper effect. While correct, it is more verbose and could be more difficult to maintain.
...
Nevertheless, this solution would be useful if the error-handling routines for each potential condition failure were different.
Noncompliant Code Example (Improper &&
)
This noncompliant code example demonstrates code that compares two arrays for ranges of members that match. Here i1
and i2
are valid array indices in array1
and array2
, respectively. It is expected that end1
and end2
will point to the end of the matching ranges in the two arrays.
...
The problem with this code is that when the first condition in the while loop fails, the second condition is not executed. That is, once i1
has reached array1.length
, the loop could terminate after i1
is executed. Consequently, the range over array1
is larger than the range over array2
, causing the final assertion to fail.
Compliant Solution (Use &
)
This compliant solution mitigates the problem by using &
, which guarantees that both i1
and i2
are incremented, regardless of the outcome of the first condition.
Code Block | ||
---|---|---|
| ||
while (++i1 < array1.length & // not && ++i2 < array2.length && array1[i1] == array2[i2]) |
Compliant Solution (Nested If Statements)
This compliant solution uses multiple if statements to achieve the proper effect. While correct, it is more verbose.
Code Block | ||
---|---|---|
| ||
while (true) { if (++i1 >= array1.length) break; if (++i2 >= array2.length) break; if (array1[i1] != array2[i2]) break; // rest of loop } |
Risk Assessment
Failure to understand the behavior of the bitwise and conditional operators can cause unintended program behavior.
Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
EXP07-J | low | unlikely | medium | P2 | L3 |
Related Guidelines
CERT C Secure Coding Standard: "EXP02-C. Be aware of the short-circuit behavior of the logical AND and OR operators"
CERT C++ Secure Coding Standard: "EXP02-CPP. Be aware of the short-circuit behavior of the logical AND and OR operators"
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="91c4f70e36920b32-cf7e555a-47824588-af178ddd-efea69e677f43b9d3a581d2e"><ac:plain-text-body><![CDATA[ | [[Flanagan 2005 | AA. Bibliography#Flanagan 05]] | 2.5.6. Boolean Operators | ]]></ac:plain-text-body></ac:structured-macro> | |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="7819b76f5bd61bfb-f8e7a9e8-4f1e4756-9d79bf84-55133ee6b6d2614d7a1ecba8"><ac:plain-text-body><![CDATA[ | [[JLS 2005 | AA. Bibliography#JLS 05]] | [§15.23, "Conditional-And Operator &&" | http://java.sun.com/docs/books/jls/third_edition/html/expressions.html#15.23] | ]]></ac:plain-text-body></ac:structured-macro> |
|
...