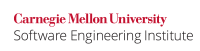
A switch
block comprises several case
labels and an optional but highly recommended default
label. Statements that follow each case
label must end with a break
statement, which is responsible for transferring the control to the end of the switch
block. When omitted, the statements in the subsequent case
label are executed. Because the break
statement is optional, omitting it produces no compiler warnings. When this behavior is unintentional, it can cause unexpected control flow.
Noncompliant Code Example
In this noncompliant code example, the case where the card
is 11 lacks a break
statement. As a result, execution continues with the statements for card = 12
.
Code Block | ||
---|---|---|
| ||
int card = 11; switch (card) { /* ... */ case 11: System.out.println("Jack"); case 12: System.out.println("Queen"); break; case 13: System.out.println("King"); break; default: System.out.println("Invalid Card"); break; } |
Compliant Solution
This compliant solution terminates each case (including the default
case) with a break
statement:
Code Block | ||
---|---|---|
| ||
int card = 11; switch (card) { /* ... */ case 11: System.out.println("Jack"); break; case 12: System.out.println("Queen"); break; case 13: System.out.println("King"); break; default: System.out.println("Invalid Card"); break; } |
Applicability
Failure to include break
statements can cause unexpected control flow.
...
Also, when a case ends with a return
or throw
statement, the break
statement may be omitted.
Bibliography
...