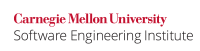
...
As a result, code that depends on the remainder operation to always return a positive result is erroneous.
Noncompliant Code Example
This noncompliant code example uses the integer hashKey
as an index into the hash
array.
...
A negative hash key produces a negative result from the remainder operator, causing the lookup()
method to throw java.lang.ArrayIndexOutOfBoundsException
.
Compliant Solution
This compliant solution calls the imod()
method that always returns a positive remainder:
Code Block | ||
---|---|---|
| ||
// Method imod() gives nonnegative result private int SIZE = 16; public int[] hash = new int[SIZE]; private int imod(int i, int j) { int temp = i % j; return (temp < 0) ? -temp : temp; // Unary - will succeed without overflow // because temp cannot be Integer.MIN_VALUE } public int lookup(int hashKey) { return hash[imod(hashKey, SIZE)]; } |
Applicability
Incorrectly assuming a positive remainder from a remainder operation can result in erroneous code.
Bibliography
...