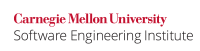
...
In this noncompliant code example, a call to System.loadLibrary()
is embedded in a doPrivileged
block. It is insecure because it could load a library on behalf of untrusted code. In essence, the untrusted code's class loader may be able to indirectly load a library even though it lacks sufficient permissions to do so directly. After loading the library, untrusted code can call native methods from the library, if those methods are accessible, because the doPrivileged
block stops any security manager checks being applied to callers further up the execution chain.
Code Block | ||
---|---|---|
| ||
public void load(String libName) {
AccessController.doPrivileged(new PrivilegedAction() {
| ||
Code Block | ||
| ||
public void load(String libName) { AccessController.doPrivileged(new PrivilegedAction() { public Object run() { System.loadLibrary(libName); return null; } }); } } |
It is insecure because it could load a library on behalf of untrusted code. In essence, the untrusted code's class loader may be able to indirectly load a library even though it lacks sufficient permissions to do so directly. After loading the library, untrusted code can call native methods from the library, if those methods are accessible, because the doPrivileged
block stops any security manager checks being applied to callers further up the execution chain.
Nonnative library code can also be susceptible to related security flaws. Loading a nonnative safe library may not directly expose a vulnerability. However, after loading an additional unsafe library, an attacker can easily exploit the safe library if it contains other vulnerabilities. Moreover, nonnative libraries often use doPrivileged
blocks, making them attractive targets.
...
This noncompliant code example returns an instance of java.sql.Connection
from trusted to untrusted code. Untrusted code that lacks the permissions required to create a SQL connection can bypass these restrictions by using the acquired instance directly.
Code Block | ||||
---|---|---|---|---|
| ||||
public Connection getConnection(String url, String username, String password) { // ... return DriverManager.getConnection(url, username, password); } |
Compliant Solution
The Untrusted code that lacks the permissions required to create a SQL connection can bypass these restrictions by using the acquired instance directly. The getConnection()
method above is unsafe because it uses the url
argument to indicate a class to be loaded; this class serves as the database driver.
Compliant Solution
This compliant solution prevents malicious users from supplying their own URL to the database connection, thereby limiting their ability to load untrusted drivers.
...
One goal of the exploit code was to access the private sun.awt.SunToolkit
class. However, invoking class.forName()
directly would cause a SecurityException
to be thrown. Consequently, the exploit code contains contained the following method to get any class, bypassing the security manager:
...