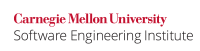
...
This compliant solution marks the fields as transient, so they are not serialized. The readObject()
method initializes them using the roll()
method. This class need not be final, as its fields are private and cannot be tampered with by subclasses, and its methods have been declared final, to prevent subclasses from overriding and ignoring them.
Code Block | ||
---|---|---|
| ||
public class Lottery implements Serializable { private transient int ticket = 1; private transient SecureRandom draw = new SecureRandom(); public Lottery(int ticket) { this.ticket = (int) (Math.abs(ticket % 20000) + 1); } public final int getTicket() { return this.ticket; } public final int roll() { this.ticket = (int) ((Math.abs(draw.nextInt()) % 20000) + 1); return this.ticket; } public static void main(String[] args) { Lottery l = new Lottery(2); for (int i = 0; i < 10; i++) { l.roll(); System.out.println(l.getTicket()); } } private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException { in.defaultReadObject(); this.draw = new SecureRandom(); roll(); } } |
...
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="82e36f34781fbc91-4a9302e7-41314a3c-84e7adf9-a98e7a5aadba6b2154957ad6"><ac:plain-text-body><![CDATA[ | [[API 2006 | AA. References#API 06]] | Class | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="9b285fbd35c8fe74-fa47716a-456248a4-b102a775-a64afb33cc5fb546867bbcb1"><ac:plain-text-body><![CDATA[ | [[Bloch 2008 | AA. References#Bloch 08]] | Item 75, Consider using a custom serialized form | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="2f21e77109b9cfd0-b2213a11-408c4733-bd67a2bf-41dbab255750a523ad75a0af"><ac:plain-text-body><![CDATA[ | [[Greanier 2000 | AA. References#Greanier 00]] |
| ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="a6223368769a82ae-1e06d1be-48514189-aa2586fd-9742f068da9e075547821c75"><ac:plain-text-body><![CDATA[ | [[Harold 1999 | AA. References#Harold 99]] | Chapter 11, Object Serialization, Validation | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="8a2c880970478580-2eaeff4f-496d439e-a69eb3f9-1371fad48a60bb738e733b63"><ac:plain-text-body><![CDATA[ | [[Hawtin 2008 | AA. References#Hawtin 08]] | Antipattern 8. Believing deserialisation is unrelated to construction | ]]></ac:plain-text-body></ac:structured-macro> |
...