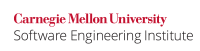
...
This noncompliant code example uses a custom-defined readObject()
method but fails to perform input validation after deserialization. The design of the system requires the maximum ticket number of any lottery ticket to be 20,000, and the ninimum ticket number be greater than 0. However, an attacker can manipulate the serialized array to generate a different number on deserialization. Such a number could be greater than 20,000, or could be 0 or negative.
Code Block | ||
---|---|---|
| ||
public class Lottery implements Serializable { private int ticket = 1; private SecureRandom draw = new SecureRandom(); public Lottery(int ticket) { this.ticket = (int) (Math.abs(ticket % 20000) + 1); } public int getTicket() { return this.ticket; } public int roll() { this.ticket = (int) ((Math.abs(draw.nextInt()) % 20000) + 1); return this.ticket; } public static void main(String[] args) { Lottery l = new Lottery(2); for (int i = 0; i < 10; i++) { l.roll(); System.out.println(l.getTicket()); } } private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException { in.defaultReadObject(); } } |
...
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="60d27d5c94aad392-559a6b42-45b24334-a2069b28-84a5a2c8e5a73a5e4065288f"><ac:plain-text-body><![CDATA[ | [[API 2006 | AA. References#API 06]] | Class | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="0f70c1790647e0eb-65e6a434-41e5497e-811ea82f-a7b3937aaaef5d54eb0ffca3"><ac:plain-text-body><![CDATA[ | [[Bloch 2008 | AA. References#Bloch 08]] | Item 75, Consider using a custom serialized form | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="564bb9d091858da1-3897da75-499540b7-87378ae5-64e6a8be43e6bcb5a26b0279"><ac:plain-text-body><![CDATA[ | [[Greanier 2000 | AA. References#Greanier 00]] |
| ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="f8bbaf7644da27f9-43de0bcb-4e6b4631-a4069504-71ced5507e0f1421af914c1a"><ac:plain-text-body><![CDATA[ | [[Harold 1999 | AA. References#Harold 99]] | Chapter 11, Object Serialization, Validation | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="f4606dad9cc84eb6-925fd655-4b70493d-86bc915b-a0f1a023880ee7d0faa8d0e9"><ac:plain-text-body><![CDATA[ | [[Hawtin 2008 | AA. References#Hawtin 08]] | Antipattern 8. Believing deserialisation is unrelated to construction | ]]></ac:plain-text-body></ac:structured-macro> |
...