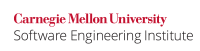
...
Do not introduce ambiguity while overloading (see 71. MET50-JG. Avoid ambiguous or confusing uses of overloading), and use overloaded methods sparingly [Tutorials 2008], because they can make code much less readable.
...
This noncompliant code example attempts to use the overloaded display()
method to perform different actions depending on whether the method is passed an ArrayList<Integer>
or a LinkedList<String>
.:
Code Block | ||
---|---|---|
| ||
public class Overloader { private static String display(ArrayList<Integer> arrayList) { return "ArrayList"; } private static String display(LinkedList<String> linkedList) { return "LinkedList"; } private static String display(List<?> list) { return "List is not recognized"; } public static void main(String[] args) { // Single ArrayList System.out.println(display(new ArrayList<Integer>())); // Array of lists List<?>[] invokeAll = new List<?>[] {new ArrayList<Integer>(), new LinkedList<String>(), new Vector<Integer>()}; for (List<?> list : invokeAll) { System.out.println(display(list)); } } } |
...
This compliant solution uses a single display
method and instanceof
to distinguish between different types. As expected, the output is ArrayList
, ArrayList
, LinkedList
, List is not recognized
.:
Code Block | ||
---|---|---|
| ||
class Overloader { public class Overloader { private static String display(List<?> list) { return ( list instanceof ArrayList ? "Arraylist" : (list instanceof LinkedList ? "LinkedList" : "List is not recognized") ); } public static void main(String[] args) { // Single ArrayList System.out.println(display(new ArrayList<Integer>())); List<?>[] invokeAll = new List<?>[] {new ArrayList<Integer>(), new LinkedList<String>(), new Vector<Integer>()}; for (List<?> list : invokeAll) { System.out.println(display(list)); } } } |
...