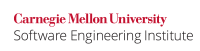
...
This noncompliant code example prints 100
as the size of the HashSet
rather than the expected result (1
). The combination of values of types short
and int
in the operation i-1
causes the result to be autoboxed into an object of type Integer
, rather than one of type Short
. The HashSet
contains only values of type Short
; the code attempts to remove objects of type Integer
. Consequently, the remove()
operation accomplishes nothing.
Code Block | ||
---|---|---|
| ||
import java.util.HashSet; public class ShortSet { public static void main(String[] args) { HashSet<Short> s = new HashSet<Short>(); for (short i = 0; i < 100; i++) { s.add(i); s.remove(i - 1); // tries to remove an Integer } System.out.println(s.size()); } } |
...
Objects removed from a collection must share the type of the elements of the collection. Numeric promotion and autoboxing can produce unexpected object types. This compliant solution uses an explicit cast to short
that matches the intended boxed type.
Code Block | ||
---|---|---|
| ||
import java.util.HashSet; public class ShortSet { public static void main(String[] args) { HashSet<Short> s = new HashSet<Short>(); for (short i = 0; i < 100; i++) { s.add(i); s.remove((short)(i - 1)); // removes a Short } System.out.println(s.size()); } } |
...
Chapter 5 | |
[JLS 2005] | |
The Joy of Sets |
02. Expressions (EXP) EXP05-J. Do not write more than once to the same variable within an expression