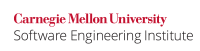
...
Code Block | ||
---|---|---|
| ||
public class Wrapper { public static void main(String[] args) { // Create an array list of integers ArrayList<Integer> list1 = new ArrayList<Integer>(); for (int i = 0; i < 10; i++) { list1.add(i + 1000); } // Create another array list of integers, where each element // has the same value as the first one ArrayList<Integer> list2 = new ArrayList<Integer>(); for (int i = 0; i < 10; i++) { list2.add(i + 1000); } // Count matching values int counter = 0; for (int i = 0; i < 10; i++) { if (list1.get(i).equals(list2.get(i))) { // uses 'equals()' counter++; } } // Print the counter: 10 in this example System.out.println(counter); } } |
Noncompliant Code Example (
...
Boolean
)
In this noncompliant code example, constructors for class Boolean
return distinct newly-instantiated objects. Using the reference equality operators in place of value comparisons will yield unexpected results.
Code Block | ||
---|---|---|
| ||
public void exampleEqualOperator(){
Boolean b1 = new Boolean("true");
Boolean b2 = new Boolean("true");
if (b1 == b2) { // never equal
System.out.println("Never printed");
}
}
|
Compliant Solution (
...
Boolean
)
In this compliant solution, the values of autoboxed Boolean
variables may be compared using the reference equality operators because the Java language guarantees that the Boolean
type is fully memoized. Consequently, these objects are guaranteed to be singletons.
Code Block | ||
---|---|---|
| ||
public void exampleEqualOperator(){ Boolean b1 = true; // Or Boolean.True Boolean b2 = true; // Or Boolean.True if (b1 == b2) { // always equal System.out.println("Always printprinted"); } } |
Risk Assessment
Using the equivalence operators to compare values of boxed primitives can lead to erroneous comparisons.
...