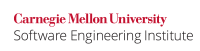
...
Code Block | ||
---|---|---|
| ||
class Point { private int x; private int y; Point(int x, int y) { this.x = x; this.y = y; } void set_xy(int x, int y) { this.x = x; this.y = y; } void print_xy() { System.out.println("the value x is: " + this.x); System.out.println("the value y is: " + this.y); } } public class PointCaller { public static void main(String[] args) { final Point point = new Point(1, 2); point.print_xy(); // Change the value of x, y point.set_xy(5, 6); point.print_xy(); } } |
When an object reference is declared final
, it signifies only that the reference cannot be changed; the mutability of the referenced object is unaffected.
Compliant Solution (final
Fields)
...
Code Block | ||
---|---|---|
| ||
class Point { private final int x; private final int y; Point(int x, int y) { this.x = x; this.y = y; } void print_xy() { System.out.println("the value x is: " + this.x); System.out.println("the value y is: " + this.y); } // Setset_xy(int x, int y) no longer possible } |
...
The Point
class is declared final
to prevent subclasses from overriding the clone()
method. This enables the class to be suitably used without any inadvertent modifications of the original object. This solution also complies with OBJ04-J. Provide mutable classes with copy functionality to safely allow passing instances to untrusted code.
Noncompliant Code Example (Arrays)
...
This compliant solution makes the array private
and provides public methods to get individual items and array size. Providing direct access to the array objects themselves is safe because String
is immutable.
Code Block | ||
---|---|---|
| ||
private static final String[] items = {/* ... */}; public static final String getItem(int index) { return items[index]; } public static final int getItemCount() { return items.length; } |
Providing direct access to the array objects themselves is safe because String
is immutable.
Compliant Solution (Clone the Array)
...
As before, this method provides direct access to the array objects themselves, which is safe because String
is immutable. If the array contained mutable objects, the getItems()
method could return a cloned an array of cloned objects.
Compliant Solution (Unmodifiable Wrappers)
...
Incorrectly assuming that final
references cause the contents of the referenced object to remain mutable can result in an attacker modifying an object thought by the programmer believed to be immutable.
Bibliography
...