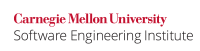
...
By providing overridden implementations, untrusted code may be able to glean sensitive information, cause arbitrary code to run and expose denial of service vulnerabilities.
See MET52-JG. Do not use the clone method to copy untrusted method parameters for more specific details regarding overriding the Object.clone()
method.
Noncompliant Code Example (hashCode)
...
Code Block | ||
---|---|---|
| ||
public class Worker implements Runnable { Worker() { } public void startThread(String name) { new Thread(this, name).start(); } @Override public void run() { System.out.println("Parent"); } } public class SubWorker extends Worker { @Override public void startThread(String name) { super.startThread(name); new Thread(this, name).start(); } @Override public void run() { System.out.println("Child"); } } |
Client If a client runs the following code:
Code Block |
---|
Worker w = new SubWorker(); w.startThread("thread"); |
The programmer expects the client may expect Parent
and Child
to be printed, however, Child
is printed twice. This is because the overridden method run()
is invoked both times when a new thread is started.
Compliant Solution
Modify This compliant solution modifies the SubWorker
class and remove removes the call to super.startThread()
.
Code Block | ||
---|---|---|
| ||
public class SubWorker extends Worker { @Override public void startThread(String name) { new Thread(this, name).start(); } // ... } |
Modify the client The client code is also modified to start the parent and child threads separately. This program produces the expected output.
Code Block |
---|
Worker w1 = new Worker(); w1.startThread("parent-thread"); Worker w2 = new SubWorker(); w2.startThread("child-thread"); |
...