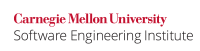
...
With the exception of the loadLibrary()
and load()
methods, the tabulated methods fail to perform any security manager checks. The loadLibrary()
and load()
APIs are typically used from within a doPrivileged
block; in that case, unprivileged callers can directly invoke them without requiring any special permissions. Consequently, the security manager checks are curtailed at the immediate caller. The security manager fails to examine the entire call stack and so fails to enhance security. Accepting tainted inputs from untrusted code and allowing them to be used by these APIs may expose vulnerabilities.
The following lists the APIs that should be used with care.
APIs that mirror language checks |
---|
|
|
|
|
|
|
|
|
Because the java.lang.reflect.Field.setAccessible/getAccessible
methods are used to instruct the JVM to override the language access checks, they perform standard (and more restrictive) security manager checks and consequently lack the vulnerability discussed in this guideline. Nevertheless, these methods should be used only with extreme caution. The remaining set*
and get*
field reflection methods perform only the language access checks and consequently are vulnerable.
Use of reflection complicates security analysis and can introduce substantial security vulnerabilities. Consequently, programmers should avoid the use of the reflection APIs when it is feasible to do so. When use of reflection is necessary, exercise extreme caution.
This guideline is an instance of SEC03-J. Do not load trusted classes after allowing untrusted code to load arbitrary classes. Many examples also violate SEC00-J. Do not allow privileged blocks to leak sensitive information across a trust boundary.
Noncompliant Code Example (CVE-2013-0422)
Java 1.7.0u10 was exploited in January 2013 because of several vulnerabilities. One vulnerability in the MBeanInstantiator
class granted unprivileged code the ability to access any class regardless of the current security policy or accessibility rules. The MBeanInstantiator.findClass()
method could be invoked with any string and would attempt to return a Class
object. This method delegated its work to the loadClass()
method, whose source code is shown:
...
This method delegates the task of dynamically loading the specified class to the Class.forName()
method. The forName()
method delegates the work of loading the class to its calling method's class loader. Since the calling method was MBeanInstantiator.loadClass()
, the core class loader is used, which provides no security checks.
Compliant Solution (CVE-2013-0422)
Oracle mitigated this vulnerability in Java 1.7.0p11 by adding an access check to the loadClass()
method. This access check ensures that the caller is permitted to access the class being sought:
Code Block | ||||
---|---|---|---|---|
| ||||
// ... if (className == null) { throw new RuntimeOperationsException(new IllegalArgumentException("The class name cannot be null"), "Exception occurred during object instantiation"); } ReflectUtil.checkPackageAccess(className); try { if (loader == null) // ... |
Noncompliant Code Example (CERT Vul# 636312)
CERT Vulnerability 636312 describes a vulnerability in Java that was successfully exploited in August 2012. (The exploit actually used two vulnerabilities; the other one is described in SEC05-J. Do not use reflection to increase accessibility of classes, methods, or fields.
...
While this method is called in the context of an applet, it uses Class.forName()
to obtain the requested class. And Class.forName()
delegates the search to the calling method's class loader. In this case the calling class (com.sun.beans.finder.ClassFinder
) is part of core Java, so the trusting class loader is used, instead of the more paranoid applet class loader.
Compliant Solution (CVE-2012-4681)
Oracle mitigated this vulnerability by patching the com.sun.beans.finder.ClassFinder.findClass()
method. The checkPackageAccess()
method checks the entire call stack to ensure that Class.forName()
in this instance only fetches classes for trusted methods.
Code Block | ||||
---|---|---|---|---|
| ||||
public static Class<?> findClass(String name) throws ClassNotFoundException { checkPackageAccess(name); try { ClassLoader loader = Thread.currentThread().getContextClassLoader(); if (loader == null) { // can be null in IE (see 6204697) loader = ClassLoader.getSystemClassLoader(); } if (loader != null) { return Class.forName(name, false, loader); } } catch (ClassNotFoundException exception) { // use current class loader instead } catch (SecurityException exception) { // use current class loader instead } return Class.forName(name); } |
Noncompliant Code Example
In this noncompliant code example a call to System.loadLibrary()
is embedded in a doPrivileged
block. This is insecure because a library can be loaded on behalf of untrusted code. In essence, the untrusted code's class loader may be able to indirectly load a library even though it lacks sufficient permissions to do so directly. After loading the library, untrusted code can call native methods from the library if those methods are accessible. This is possible because the doPrivileged
block stops security manager checks being applied to callers further up the execution chain.
...
Nonnative library code can also be susceptible to related security flaws. Loading a nonnative safe library may not directly expose a vulnerability. However, after loading an additional unsafe library, an attacker can easily exploit the safe library if it contains other vulnerabilities. Moreover, nonnative libraries often use doPrivileged
blocks, making them attractive targets.
Compliant Solution
This compliant solution hard-codes the name of the library to prevent the possibility of tainted values. It also reduces the accessibility of method load()
from public
to private
. Consequently, untrusted callers are prohibited from loading the awt
library.
Code Block | ||
---|---|---|
| ||
private void load() { AccessController.doPrivileged(new PrivilegedAction() { public Object run() { System.loadLibrary("awt"); return null; } }); } |
Noncompliant Code Example
A method that passes untrusted inputs to the Class.forName()
method might permit an attacker to access classes with escalated privileges. The single argument Class.forName()
method is another API method that uses its immediate caller's class loader to load a requested class. Untrusted code can misuse this API to indirectly manufacture classes that have the same privileges as those of the attacker's immediate caller.
Code Block | ||||
---|---|---|---|---|
| ||||
public Class loadClass(String className) { // className may be the name of a privileged or even a malicious class return Class.forName(className); } |
Compliant Solution
This compliant solution hard-codes the class's name.
Code Block | ||||
---|---|---|---|---|
| ||||
public Class loadClass() { return Class.forName("Foo"); } |
Noncompliant Code Example
This noncompliant code example returns an instance of java.sql.Connection
from trusted to untrusted code. Untrusted code that lacks the permissions required to create a SQL connection can bypass these restrictions by using the acquired instance directly.
Code Block | ||||
---|---|---|---|---|
| ||||
public Connection getConnection(String url, String username, String password) { // ... return DriverManager.getConnection(url, username, password); } |
Compliant Solution
The getConnection()
method is unsafe because it uses the url
to indicate a class to be loaded; this class serves as the database driver. This compliant solution prevents a malicious user from supplying their own URL to the database connection; thereby limiting their ability to load untrusted drivers.
Code Block | ||||
---|---|---|---|---|
| ||||
private String url = // hardwired value public Connection getConnection(String username, String password) { // ... return DriverManager.getConnection(this.url, username, password); } |
Applicability
Allowing untrusted code to carry out actions using the immediate caller's class loader may allow the untrusted code to execute with the same privileges as the immediate caller.
...
Code Block |
---|
public static Class forName(String name, boolean initialize, ClassLoader loader) /* explicitly specify the class loader to use */ throws ClassNotFoundException |
Related Guidelines
[SCG 2010] | Guideline 9-9: Safely invoke standard APIs that perform tasks using the immediate caller's class loader instance |
Bibliography
...