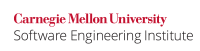
...
Code Block | ||
---|---|---|
| ||
final class ControlledStop implements Runnable { private volatile boolean done = false; public void run() { while (!done) { try { // ... Thread.currentThread().sleep(1000); // Do something } catch(InterruptedException ie) { // handle exception } } } protected void shutdown(){ done = true; } } |
Exceptions
EX1: Correctly synchronized code that accesses a shared primitive variable always observes its latest value. In this case there is no requirement of explicitly ensuring visibility. However, excessive synchronization can lead to lock contention and deadlocks. Consequently, it should not be used unless there is some other need to use it, than using it to ensure the visibility of accesses to shared primitive variables. This exception performs operations that require synchronization (incrementing i
) and as a side-effect, it ensures the visibility of the done
flag.
Code Block | ||
---|---|---|
| ||
final class ControlledStop implements Runnable {
int i = 0;
private boolean done = false;
public synchronized void run() {
while (!done) {
// Do something
i++;
}
}
protected void shutdown(){
done = true;
}
}
|
Risk Assessment
Failing to ensure visibility of atomically modifiable shared variables can lead to a thread seeing stale values of a variable.
...